The Java String isEmpty function is a built-in method that checks whether a given string is empty or not. It returns a boolean value of true if the string is empty, i.e., it contains no characters, and false if it contains one or more characters. The function is useful in scenarios where we need to validate user input or check if a string variable has been initialized with a value. It is a simple and efficient way to check for empty strings without having to write custom code. Keep reading below to learn how to Java String isEmpty in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
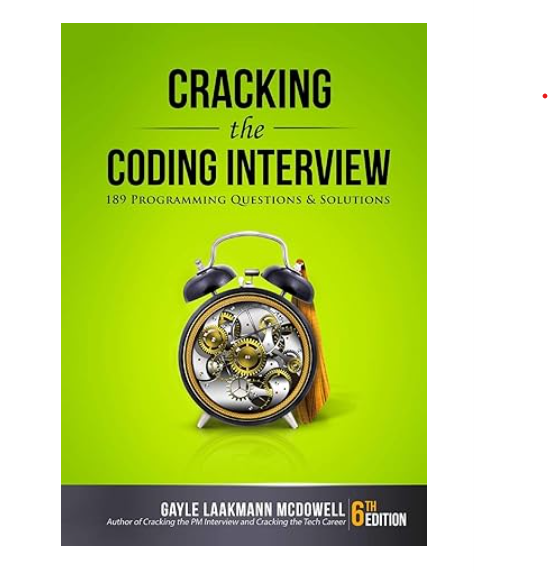
Java String isEmpty in C++ With Example Code
Java’s String class has a method called `isEmpty()` that checks if a string is empty or not. However, C++ does not have a built-in method for this. In this blog post, we will discuss how to implement the `isEmpty()` method in C++.
To check if a string is empty in C++, we can use the `empty()` method. This method returns a boolean value indicating whether the string is empty or not. Here’s an example:
#include
#include
using namespace std;
int main() {
string str1 = "";
string str2 = "Hello, world!";
if (str1.empty()) {
cout << "str1 is empty" << endl;
} else {
cout << "str1 is not empty" << endl;
}
if (str2.empty()) {
cout << "str2 is empty" << endl;
} else {
cout << "str2 is not empty" << endl;
}
return 0;
}
In this example, we create two strings `str1` and `str2`. `str1` is empty, while `str2` contains the string "Hello, world!". We then use the `empty()` method to check if each string is empty or not. The output of this program will be:
str1 is empty
str2 is not empty
As you can see, the `empty()` method returns `true` if the string is empty and `false` if it is not.
In conclusion, while C++ does not have a built-in `isEmpty()` method like Java's String class, we can use the `empty()` method to achieve the same functionality.
Equivalent of Java String isEmpty in C++
In conclusion, the equivalent function to Java's String isEmpty() in C++ is the empty() function. Both functions serve the same purpose of checking whether a string is empty or not. However, it is important to note that the syntax and usage of these functions differ slightly between the two programming languages. While Java's String isEmpty() function returns a boolean value, C++'s empty() function returns a boolean expression. Nonetheless, both functions are essential in ensuring that strings are not manipulated when they are empty, which can lead to errors and bugs in the program. Therefore, it is important for programmers to understand the equivalent functions in different programming languages to ensure that their code is efficient and error-free.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |