The Java String isEmpty function is a built-in method that checks whether a given string is empty or not. It returns a boolean value of true if the string is empty, i.e., it contains no characters, and false if it contains one or more characters. The function is useful in scenarios where we need to validate user input or check if a string variable has been initialized with a value. It is a simple and efficient way to check for empty strings without having to write custom code. Keep reading below to learn how to Java String isEmpty in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
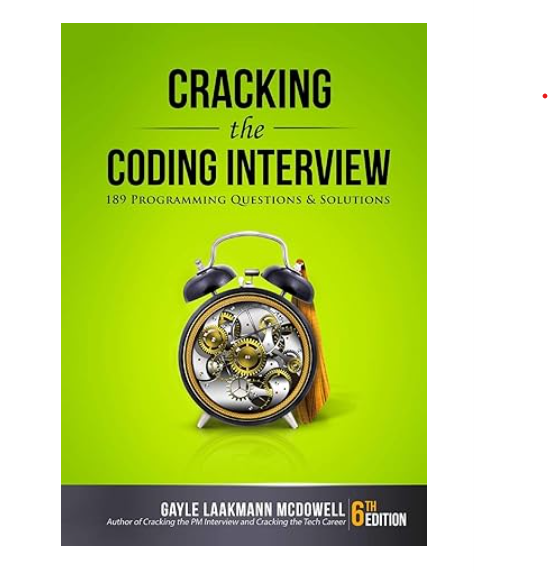
Java String isEmpty in PHP With Example Code
Java String isEmpty() is a method that checks whether a string is empty or not. In PHP, we can use the empty() function to check if a string is empty or not. The empty() function returns true if the variable is empty, and false otherwise.
To check if a string is empty in PHP, we can use the empty() function as follows:
if(empty($string)) {
echo "String is empty";
} else {
echo "String is not empty";
}
In the above code, we are checking if the $string variable is empty or not using the empty() function. If the $string variable is empty, we are printing “String is empty”, otherwise we are printing “String is not empty”.
We can also use the strlen() function to check if a string is empty or not. The strlen() function returns the length of the string. If the length of the string is 0, then the string is empty.
if(strlen($string) == 0) {
echo "String is empty";
} else {
echo "String is not empty";
}
In the above code, we are checking if the length of the $string variable is 0 or not using the strlen() function. If the length of the $string variable is 0, we are printing “String is empty”, otherwise we are printing “String is not empty”.
In conclusion, we can use the empty() function or the strlen() function to check if a string is empty or not in PHP.
Equivalent of Java String isEmpty in PHP
In conclusion, the equivalent function of Java’s String isEmpty() in PHP is the empty() function. Both functions serve the same purpose of checking if a string is empty or not. However, it is important to note that the empty() function in PHP has a broader scope as it can also check if variables are empty, not just strings. Therefore, when working with PHP, it is recommended to use the empty() function to check for empty strings or variables. By using the correct function, you can ensure that your code is efficient and effective in achieving its intended purpose.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |