The Java String isEmpty function is a built-in method that checks whether a given string is empty or not. It returns a boolean value of true if the string is empty, i.e., it contains no characters, and false if it contains one or more characters. The function is useful in scenarios where we need to validate user input or check if a string variable has been initialized with a value. It is a simple and efficient way to check for empty strings without having to write custom code. Keep reading below to learn how to Java String isEmpty in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
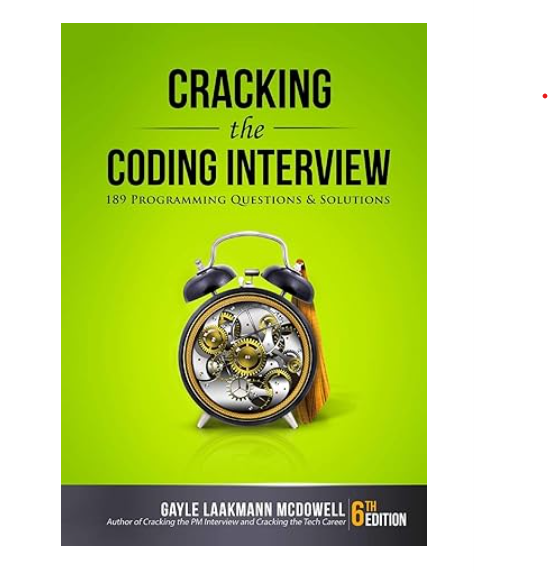
Java String isEmpty in Rust With Example Code
Java’s String class has a convenient method called `isEmpty()` that checks if a string is empty or not. Rust, on the other hand, does not have a built-in method for this. However, it is easy to implement this functionality using Rust’s standard library.
To check if a string is empty in Rust, we can simply check its length. If the length is zero, then the string is empty. Here’s an example code snippet that demonstrates this:
fn is_empty(s: &str) -> bool {
s.len() == 0
}
In this code, we define a function called `is_empty` that takes a string slice (`&str`) as an argument and returns a boolean value indicating whether the string is empty or not. The function simply checks the length of the string using the `len()` method and returns `true` if the length is zero.
We can use this function to check if a string is empty like this:
let s = "";
if is_empty(s) {
println!("The string is empty");
} else {
println!("The string is not empty");
}
In this example, we define a string `s` that is empty. We then call the `is_empty()` function with `s` as an argument to check if it is empty or not. If it is empty, we print a message saying so. Otherwise, we print a message saying that it is not empty.
In conclusion, while Rust does not have a built-in method for checking if a string is empty, it is easy to implement this functionality using Rust’s standard library. By checking the length of a string, we can determine if it is empty or not.
Equivalent of Java String isEmpty in Rust
In conclusion, the Rust programming language provides a simple and efficient way to check if a string is empty using the `is_empty()` function. This function is equivalent to the Java `isEmpty()` function and returns a boolean value indicating whether the string is empty or not. By using this function, Rust developers can easily check if a string is empty and take appropriate actions based on the result. Overall, the `is_empty()` function is a useful tool for Rust developers who want to write clean and concise code that is easy to read and maintain.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |