The Java String join function is a method that allows you to concatenate multiple strings into a single string, using a specified delimiter. It takes an array or an iterable of strings as input, along with the delimiter that you want to use to separate the strings. The method then joins the strings together, inserting the delimiter between each string, and returns the resulting string. This function is useful when you need to combine multiple strings into a single string, such as when constructing a message or a file path. Keep reading below to learn how to Java String join in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
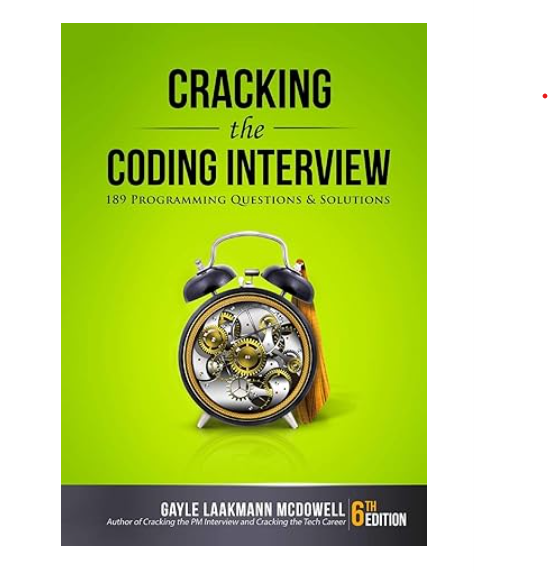
Java String join in C++ With Example Code
Java has a built-in method called `String.join()` that allows you to concatenate multiple strings with a delimiter. Unfortunately, C++ does not have a built-in equivalent. However, there are a few ways to achieve the same result.
One way is to use `std::stringstream`. This class allows you to write to a string as if it were a stream. You can use the `<<` operator to write each string to the stream, separated by the delimiter. Then, you can retrieve the final string using the `str()` method.
Here's an example:
#include
#include
#include
std::string join(const std::vector
std::stringstream ss;
for (size_t i = 0; i < strings.size(); ++i) {
if (i != 0) {
ss << delimiter;
}
ss << strings[i];
}
return ss.str();
}
int main() {
std::vector
std::string delimiter = “, “;
std::string result = join(strings, delimiter);
std::cout << result << std::endl; // prints "foo, bar, baz"
return 0;
}
In this example, the `join()` function takes a vector of strings and a delimiter as arguments. It uses a `std::stringstream` to concatenate the strings with the delimiter. Finally, it returns the resulting string.
Another way to achieve the same result is to use `std::accumulate()`. This function is part of the C++ standard library and allows you to accumulate a range of values into a single value. You can use it to concatenate the strings with the delimiter.
Here’s an example:
#include
#include
#include
std::string join(const std::vector
return std::accumulate(strings.begin(), strings.end(), std::string(),
[&](const std::string& a, const std::string& b) -> std::string {
return a.empty() ? b : a + delimiter + b;
});
}
int main() {
std::vector
std::string delimiter = ", ";
std::string result = join(strings, delimiter);
std::cout << result << std::endl; // prints "foo, bar, baz"
return 0;
}
In this example, the `join()` function uses `std::accumulate()` to concatenate the strings with the delimiter. The lambda function passed to `std::accumulate()` checks if the accumulated string is empty. If it is, it returns the current string. Otherwise, it concatenates the current string with the delimiter and the accumulated string.
Both of these methods allow you to achieve the same result as `String.join()` in Java.
Equivalent of Java String join in C++
In conclusion, while Java has a built-in String join function, C++ does not have an equivalent function in its standard library. However, there are several ways to achieve the same functionality in C++, such as using the stringstream class or creating a custom function using iterators and the std::accumulate function. It is important to consider the specific requirements of your project and choose the method that best suits your needs. With a little bit of creativity and knowledge of C++ syntax, you can easily replicate the functionality of Java's String join function in your C++ code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |