The Java String join function is a method that allows you to concatenate multiple strings into a single string, using a specified delimiter. It takes an array or an iterable of strings as input, along with the delimiter that you want to use to separate the strings. The join function then combines all the strings in the array or iterable, inserting the delimiter between each pair of strings. The resulting string is returned as the output of the function. This function is useful for creating formatted output or for constructing complex strings from multiple smaller strings. Keep reading below to learn how to Java String join in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
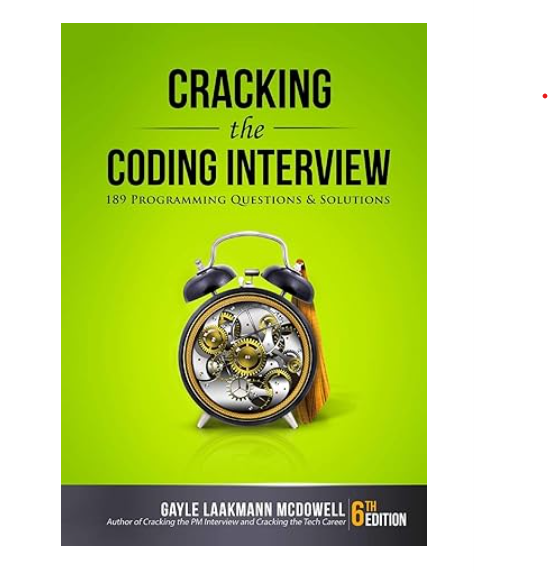
Java String join in TypeScript With Example Code
Java String join is a useful method that allows you to concatenate a list of strings with a delimiter. TypeScript, being a superset of JavaScript, also provides a similar method to join strings.
To join strings in TypeScript, you can use the `join()` method of an array. This method takes a delimiter as an argument and returns a string that is the concatenation of all the elements in the array, separated by the delimiter.
Here’s an example:
const fruits = ['apple', 'banana', 'orange'];
const joinedFruits = fruits.join(', ');
console.log(joinedFruits); // Output: "apple, banana, orange"
In this example, we have an array of fruits and we use the `join()` method to join them with a comma and a space as the delimiter.
You can also use the `join()` method with a template literal to join strings with a custom separator. Here’s an example:
const name = 'John';
const age = 30;
const address = '123 Main St';
const userDetails = [name, age, address].join(`\n`);
console.log(userDetails);
// Output:
// John
// 30
// 123 Main St
In this example, we have an array of user details and we use the `join()` method with a template literal to join them with a newline character as the delimiter.
In conclusion, the `join()` method in TypeScript is a simple and effective way to join strings with a delimiter. It can be used with arrays and template literals to create custom separators.
Equivalent of Java String join in TypeScript
In conclusion, TypeScript provides a powerful and efficient way to join strings using the `join()` function. This function works similarly to the equivalent Java String join function, allowing developers to easily concatenate strings with a specified delimiter. By using this function, developers can write cleaner and more concise code, making it easier to read and maintain. Additionally, TypeScript’s strong typing system ensures that the function is used correctly, reducing the likelihood of errors and bugs. Overall, the `join()` function is a valuable tool for any TypeScript developer looking to streamline their string manipulation code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |