The Java String matches function is a method that checks whether a string matches a specified regular expression. It returns a boolean value indicating whether the entire string matches the regular expression or not. The regular expression is a pattern that defines a set of strings, and the matches function compares the input string with this pattern. If the input string matches the pattern, the function returns true; otherwise, it returns false. The matches function is useful for validating user input, searching for specific patterns in a string, and manipulating strings based on certain criteria. Keep reading below to learn how to Java String matches in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
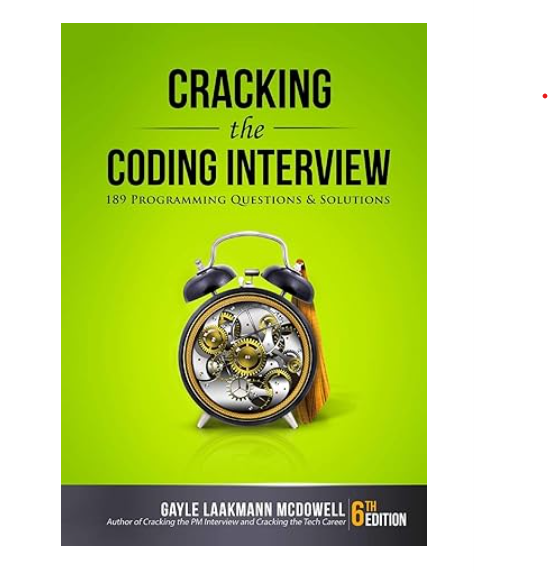
Java String matches in Rust With Example Code
Java String matches in Rust can be achieved using the regex crate. This crate provides a way to work with regular expressions in Rust. To use the regex crate, add it to your Cargo.toml file:
[dependencies]
regex = "1.5.4"
Once you have added the regex crate to your project, you can use it to match strings. Here is an example:
use regex::Regex;
fn main() {
let re = Regex::new(r"^\d{4}-\d{2}-\d{2}$").unwrap();
assert!(re.is_match("2021-10-01"));
}
In this example, we create a regular expression that matches a date in the format “YYYY-MM-DD”. We then use the is_match method to check if a given string matches the regular expression.
You can also use the find method to find the first occurrence of a regular expression in a string:
use regex::Regex;
fn main() {
let re = Regex::new(r"\d+").unwrap();
let text = "Rust 1.55 was released on 2021-08-26";
if let Some(matched) = re.find(text) {
println!("Matched: {}", matched.as_str());
}
}
In this example, we create a regular expression that matches one or more digits. We then use the find method to find the first occurrence of the regular expression in the string “Rust 1.55 was released on 2021-08-26”.
Overall, using the regex crate in Rust provides a powerful way to work with regular expressions and match strings.
Equivalent of Java String matches in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to handle string matching with its built-in regular expression library. The equivalent Java String matches function can be easily replicated in Rust using the Regex crate. With its simple syntax and robust functionality, Rust’s Regex library makes it easy to perform complex string matching operations in a concise and efficient manner. Whether you are a seasoned Rust developer or just getting started with the language, the Regex crate is a valuable tool to have in your toolkit for working with strings. So, if you’re looking for a reliable and efficient way to handle string matching in Rust, look no further than the Regex crate.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |