The Java String matches function is a method that checks whether a string matches a specified regular expression. It returns a boolean value indicating whether the entire string matches the regular expression or not. The regular expression is a pattern that defines a set of strings, and the matches function compares the input string with this pattern. If the input string matches the pattern, the function returns true; otherwise, it returns false. The matches function is useful for validating user input, searching for specific patterns in a string, and manipulating strings based on certain criteria. Keep reading below to learn how to Java String matches in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
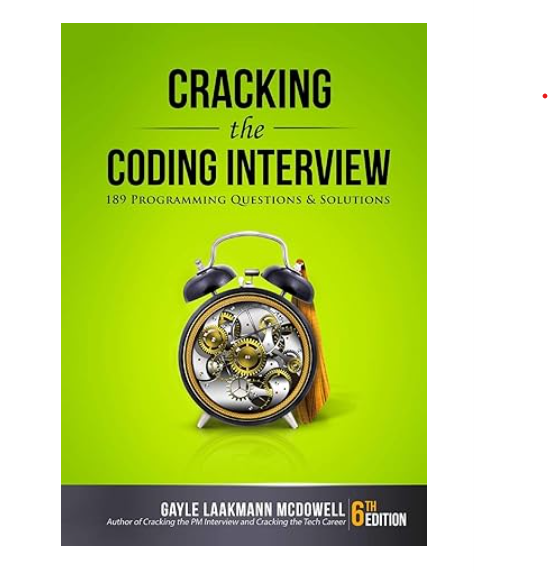
Java String matches in TypeScript With Example Code
Java String matches is a useful method for checking if a string matches a regular expression. In TypeScript, we can use the same method with a few minor differences.
To use the matches method in TypeScript, we first need to create a regular expression object. We can do this by using the RegExp constructor or by using a regular expression literal.
const regex = new RegExp('pattern');
const regexLiteral = /pattern/;
Once we have our regular expression object, we can use the matches method on a string to check if it matches the pattern.
const str = 'example string';
const regex = /example/;
const matches = str.match(regex);
console.log(matches); // ['example']
The matches method returns an array of all the matches found in the string. If no matches are found, it returns null.
We can also use the matches method with capture groups in our regular expression.
const str = 'example string';
const regex = /(example) (string)/;
const matches = str.match(regex);
console.log(matches); // ['example string', 'example', 'string']
In this example, we have two capture groups in our regular expression. The matches method returns an array with the full match as the first element, followed by the matches for each capture group.
In conclusion, the matches method in TypeScript works the same way as in Java. We just need to create a regular expression object and use the matches method on a string to check if it matches the pattern.
Equivalent of Java String matches in TypeScript
In conclusion, the equivalent function of Java’s String matches() in TypeScript is the match() function. Both functions are used to match a regular expression pattern against a string and return a boolean value indicating whether the pattern matches the string or not. However, there are some differences between the two functions. For instance, the match() function in TypeScript returns an array of matches if the global flag is set, while the matches() function in Java returns a boolean value indicating whether the entire string matches the pattern or not. Additionally, the match() function in TypeScript is case-sensitive by default, while the matches() function in Java is case-insensitive by default. Therefore, it is important to understand these differences when using the match() function in TypeScript to ensure that it meets your specific needs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |