The Java String replace function is used to replace all occurrences of a specified character or substring within a given string with a new character or substring. It takes two parameters: the first parameter is the character or substring to be replaced, and the second parameter is the new character or substring that will replace the old one. The function returns a new string with all the replacements made. If the specified character or substring is not found in the original string, the function returns the original string unchanged. The replace function is a useful tool for manipulating strings in Java programs. Keep reading below to learn how to Java String replace in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
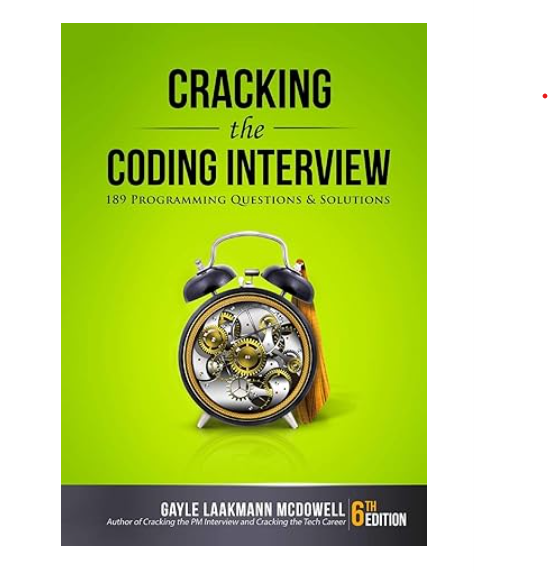
Java String replace in Rust With Example Code
Java is a popular programming language that is widely used for developing various applications. One of the common tasks in Java programming is replacing a string with another string. Rust is another programming language that is gaining popularity due to its performance and memory safety features. In this blog post, we will discuss how to replace a string in Rust.
To replace a string in Rust, we can use the `replace` method provided by the `String` type. The `replace` method takes two arguments: the string to be replaced and the string to replace it with. Here is an example code snippet that demonstrates how to use the `replace` method:
let original_string = "Hello, world!";
let new_string = original_string.replace("world", "Rust");
println!("{}", new_string);
In the above code, we first define a string `original_string` that contains the text “Hello, world!”. We then use the `replace` method to replace the word “world” with “Rust” and store the result in a new string `new_string`. Finally, we print the value of `new_string` to the console.
It is important to note that the `replace` method returns a new string and does not modify the original string. If we want to modify the original string, we can use the `replace_range` method provided by the `String` type. The `replace_range` method takes two arguments: the range of the string to be replaced and the string to replace it with. Here is an example code snippet that demonstrates how to use the `replace_range` method:
let mut original_string = String::from("Hello, world!");
let new_string = String::from("Rust");
original_string.replace_range(7..12, &new_string);
println!("{}", original_string);
In the above code, we first define a mutable string `original_string` that contains the text “Hello, world!”. We then define a new string `new_string` that contains the text “Rust”. We use the `replace_range` method to replace the range of characters from index 7 to index 12 (exclusive) with the value of `new_string`. Finally, we print the value of `original_string` to the console.
In conclusion, replacing a string in Rust is a simple task that can be accomplished using the `replace` or `replace_range` method provided by the `String` type. These methods provide a convenient way to modify strings in Rust without having to write complex code.
Equivalent of Java String replace in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to replace substrings within a string using the `replace` function. This function works similarly to the Java `replace` function, allowing developers to easily manipulate strings in their Rust programs. With its focus on safety, performance, and concurrency, Rust is quickly becoming a popular choice for developers who want to build fast and reliable applications. Whether you are a seasoned developer or just starting out, Rust’s `replace` function is a valuable tool to have in your programming arsenal. So why not give it a try and see how it can help you build better software today?
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |