The Java String replace function is a method that allows you to replace all occurrences of a specified character or substring within a string with a new character or substring. It takes two parameters: the first parameter is the character or substring to be replaced, and the second parameter is the character or substring to replace it with. The method returns a new string with all occurrences of the specified character or substring replaced with the new character or substring. This function is useful for manipulating strings and making changes to specific parts of a string without having to manually search and replace each occurrence. Keep reading below to learn how to Java String replace in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
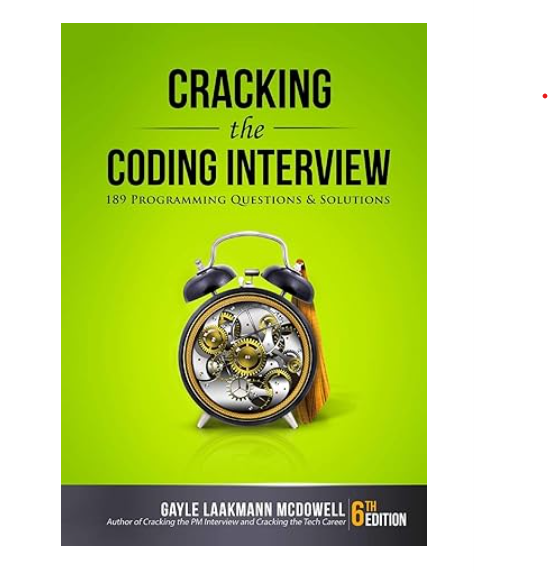
Java String replace in TypeScript With Example Code
Java String replace method is a commonly used method in Java programming. However, if you are working with TypeScript, you may wonder how to use this method in your TypeScript code. In this blog post, we will discuss how to Java String replace in TypeScript.
To use the Java String replace method in TypeScript, you can simply use the replace method of the string object. The replace method takes two parameters: the string to be replaced and the string to replace it with. Here is an example code snippet:
let str: string = "Hello World!";
let newStr: string = str.replace("World", "TypeScript");
console.log(newStr); // Output: "Hello TypeScript!"
In the above example, we have used the replace method to replace the string “World” with “TypeScript” in the original string “Hello World!”.
You can also use regular expressions with the replace method to replace multiple occurrences of a string. Here is an example code snippet:
let str: string = "Hello World! Hello TypeScript!";
let newStr: string = str.replace(/Hello/g, "Hi");
console.log(newStr); // Output: "Hi World! Hi TypeScript!"
In the above example, we have used a regular expression with the replace method to replace all occurrences of the string “Hello” with “Hi” in the original string “Hello World! Hello TypeScript!”.
In conclusion, using the Java String replace method in TypeScript is as simple as using the replace method of the string object. You can also use regular expressions with the replace method to replace multiple occurrences of a string.
Equivalent of Java String replace in TypeScript
In conclusion, TypeScript provides a powerful and efficient way to replace strings using the `replace()` function. This function works similarly to the equivalent Java String replace function, allowing developers to easily replace specific characters or patterns within a string. With TypeScript’s strong typing and object-oriented features, developers can write cleaner and more maintainable code when working with strings. Whether you’re building a web application or a mobile app, TypeScript’s `replace()` function is a valuable tool to have in your toolkit. So, if you’re looking for a reliable and efficient way to replace strings in your TypeScript code, look no further than the `replace()` function.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |