The Java String replaceAll function is a method that replaces all occurrences of a specified regular expression with a given replacement string. It takes two arguments: the first argument is the regular expression to be replaced, and the second argument is the replacement string. The method searches the input string for all occurrences of the regular expression and replaces them with the replacement string. The resulting string is then returned. This method is useful for manipulating strings and can be used in a variety of applications, such as data cleaning and text processing. Keep reading below to learn how to Java String replaceAll in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
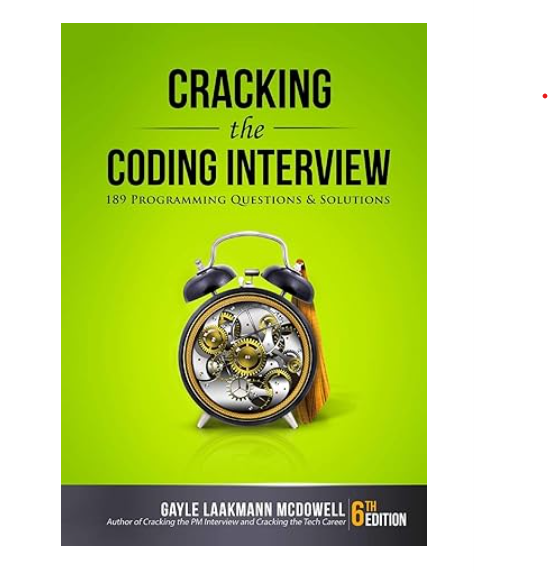
Java String replaceAll in C# With Example Code
Java’s `String.replaceAll()` method is a powerful tool for replacing substrings within a string. However, if you’re working in C#, you may be wondering how to achieve the same functionality. Fortunately, C# provides a similar method called `Regex.Replace()`.
To use `Regex.Replace()`, you’ll need to import the `System.Text.RegularExpressions` namespace. Once you’ve done that, you can call the method on a string and pass in a regular expression pattern to match the substring(s) you want to replace. You can also pass in a replacement string to specify what to replace the matched substring(s) with.
Here’s an example of how to use `Regex.Replace()` in C#:
using System.Text.RegularExpressions;
string originalString = "The quick brown fox jumps over the lazy dog.";
string pattern = "fox";
string replacement = "cat";
string newString = Regex.Replace(originalString, pattern, replacement);
Console.WriteLine(newString); // Output: "The quick brown cat jumps over the lazy dog."
In this example, we’re replacing the substring “fox” with “cat” in the original string. The resulting string is then printed to the console.
Overall, `Regex.Replace()` provides a powerful and flexible way to replace substrings in C#.
Equivalent of Java String replaceAll in C#
In conclusion, the equivalent function to Java’s String replaceAll in C# is the Regex.Replace method. This method allows for the replacement of all occurrences of a specified pattern in a string with a new value. While the syntax may differ slightly between the two languages, the functionality remains the same. By using Regex.Replace in C#, developers can easily manipulate strings and perform complex pattern matching operations. Overall, understanding the similarities and differences between these two functions can help developers write more efficient and effective code in both Java and C#.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |