The Java String replaceAll function is a method that replaces all occurrences of a specified regular expression with a given replacement string. It takes two arguments: the first argument is the regular expression to be replaced, and the second argument is the replacement string. The method searches the entire string and replaces all occurrences of the regular expression with the replacement string. The replaceAll function returns a new string with the replaced values. This method is useful for manipulating strings and can be used in various applications such as data cleaning, text processing, and data validation. Keep reading below to learn how to Java String replaceAll in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
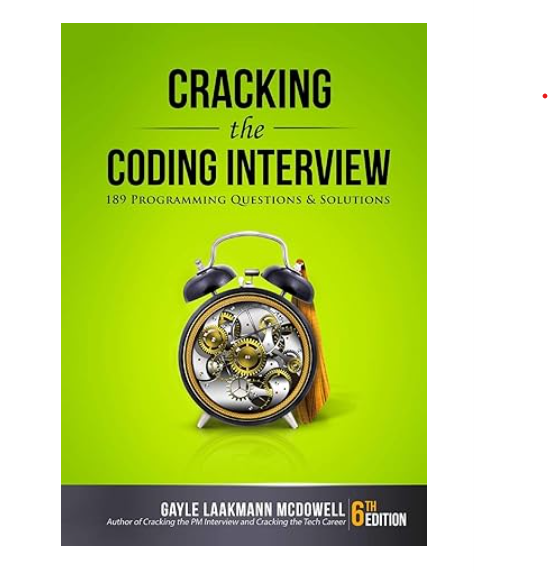
Java String replaceAll in Rust With Example Code
Java’s `String.replaceAll()` method is a powerful tool for replacing substrings within a string. If you’re working in Rust and need to perform similar operations, fear not! Rust has its own set of string manipulation methods that can accomplish the same task.
One of the most commonly used methods for replacing substrings in Rust is `replace()`. This method takes two arguments: the substring to be replaced, and the replacement string. Here’s an example:
let my_string = "Hello, world!";
let new_string = my_string.replace("world", "Rust");
println!("{}", new_string);
This code will output “Hello, Rust!”.
If you need to replace multiple occurrences of a substring, you can use the `replace_all()` method. This method works similarly to `replace()`, but replaces all occurrences of the substring instead of just the first one. Here’s an example:
let my_string = "Hello, world! Hello, Rust!";
let new_string = my_string.replace_all("Hello", "Hi");
println!("{}", new_string);
This code will output “Hi, world! Hi, Rust!”.
In addition to `replace()` and `replace_all()`, Rust also has a number of other string manipulation methods that can be useful in different situations. Some of these methods include `trim()`, `to_lowercase()`, and `to_uppercase()`. By familiarizing yourself with these methods, you’ll be well-equipped to handle any string manipulation tasks that come your way in Rust.
Equivalent of Java String replaceAll in Rust
In conclusion, Rust provides a powerful and efficient way to replace all occurrences of a substring in a string using the `replace_all` function. This function is similar to the `replaceAll` function in Java and allows developers to easily manipulate strings in their Rust programs. With its simple syntax and high performance, Rust’s `replace_all` function is a great tool for any developer looking to work with strings in their Rust projects. Whether you’re a seasoned Rust developer or just getting started, the `replace_all` function is a valuable addition to your toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |