The Java String replaceFirst function is a method that allows you to replace the first occurrence of a specified regular expression in a given string with a new string. The method takes two arguments: the regular expression to be replaced and the replacement string. If the regular expression is found in the string, the first occurrence is replaced with the replacement string and the resulting string is returned. If the regular expression is not found in the string, the original string is returned unchanged. This method is useful for making specific changes to a string without affecting other occurrences of the same regular expression. Keep reading below to learn how to Java String replaceFirst in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
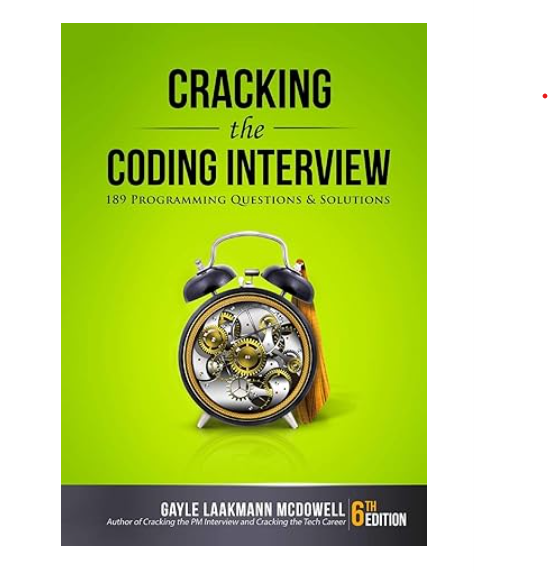
Java String replaceFirst in Python With Example Code
Java and Python are two popular programming languages used for various purposes. While they have many similarities, they also have some differences in syntax and functionality. One of the differences is the way they handle string manipulation. In Java, there is a method called replaceFirst() that allows you to replace the first occurrence of a substring in a string. In Python, there is no direct equivalent to this method, but there are several ways to achieve the same result.
To replace the first occurrence of a substring in a string using Java’s replaceFirst() method, you can use the following syntax:
String newString = originalString.replaceFirst("oldSubstring", "newSubstring");
This will replace the first occurrence of “oldSubstring” in the “originalString” with “newSubstring” and store the result in “newString”.
In Python, one way to achieve the same result is to use the replace() method with a maximum number of replacements specified. By default, the replace() method replaces all occurrences of a substring in a string. However, you can limit the number of replacements by specifying a maximum number as the third argument. To replace only the first occurrence of a substring, you can set the maximum number to 1. Here’s an example:
new_string = original_string.replace("old_substring", "new_substring", 1)
This will replace the first occurrence of “old_substring” in the “original_string” with “new_substring” and store the result in “new_string”.
Another way to achieve the same result in Python is to use regular expressions with the re module. Here’s an example:
import re
new_string = re.sub(r'old_substring', 'new_substring', original_string, 1)
This will replace the first occurrence of “old_substring” in the “original_string” with “new_substring” using regular expressions and store the result in “new_string”.
In conclusion, while Java’s replaceFirst() method and Python’s replace() method have some differences in syntax and functionality, there are several ways to achieve the same result in Python using either the replace() method with a maximum number of replacements specified or regular expressions with the re module.
Equivalent of Java String replaceFirst in Python
In conclusion, the equivalent function of Java’s replaceFirst() in Python is the re.sub() method. This method allows you to replace the first occurrence of a pattern in a string with a specified replacement string. It is a powerful tool that can be used to manipulate strings in Python, and it is very similar to the replaceFirst() function in Java. By using the re.sub() method, you can easily replace the first occurrence of a pattern in a string, making it a valuable addition to your Python programming toolkit. So, if you are looking for a way to replace the first occurrence of a pattern in a string in Python, the re.sub() method is the way to go.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |