The Java String split function is a method that allows a string to be split into an array of substrings based on a specified delimiter. The split function takes a regular expression as its argument, which is used to identify the delimiter. The resulting array contains all the substrings that were separated by the delimiter. The split function can be useful for parsing text data or breaking down a string into smaller components. It is commonly used in Java programming for data manipulation and processing. Keep reading below to learn how to Java String split in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
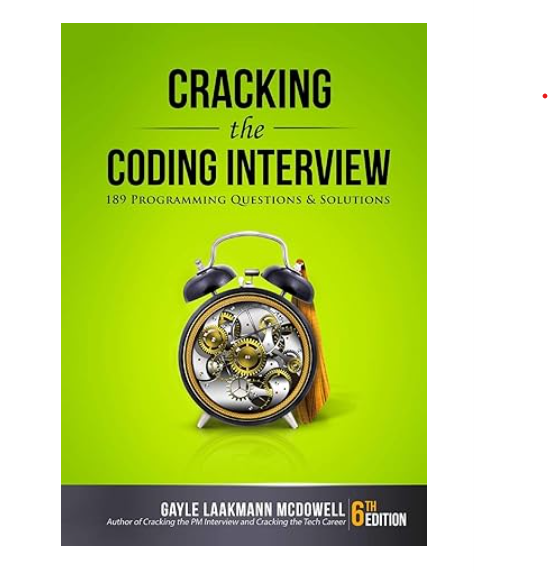
Java String split in C# With Example Code
Java String split() method is a very useful method when it comes to splitting a string into an array of substrings based on a delimiter. However, if you are working with C#, you might be wondering how to achieve the same functionality. In this blog post, we will explore how to Java String split in C#.
In C#, the equivalent method to Java’s split() method is the Split() method of the String class. This method takes in a character array as a delimiter and returns an array of substrings.
Here is an example of how to use the Split() method in C#:
string str = "Hello,World";
char[] delimiter = { ',' };
string[] substrings = str.Split(delimiter);
foreach (string substring in substrings)
{
Console.WriteLine(substring);
}
In the above example, we first define a string “Hello,World”. We then define a character array containing the delimiter (‘,’). We then call the Split() method on the string and pass in the delimiter array. The method returns an array of substrings, which we then loop through and print out each substring.
It is important to note that the Split() method in C# is case-sensitive. If you want to perform a case-insensitive split, you can use the Split() method overload that takes in a StringComparison parameter.
In conclusion, the Split() method in C# provides similar functionality to Java’s split() method. By using the Split() method, you can easily split a string into an array of substrings based on a delimiter.
Equivalent of Java String split in C#
In conclusion, the equivalent Java String split function in C# is the Split() method. This method allows developers to split a string into an array of substrings based on a specified delimiter. The Split() method in C# is similar to the split() function in Java, but with some minor differences in syntax and functionality. However, both methods serve the same purpose of breaking down a string into smaller parts for easier manipulation and analysis. As a developer, it is important to understand the similarities and differences between these two methods to effectively use them in your code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |