The Java String split function is a method that allows a string to be split into an array of substrings based on a specified delimiter. The split function takes a regular expression as its argument, which is used to identify the delimiter. The resulting array contains all the substrings that were separated by the delimiter. The split function can be useful for parsing text data or breaking down a string into smaller components. It is commonly used in Java programming for data manipulation and processing. Keep reading below to learn how to Java String split in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
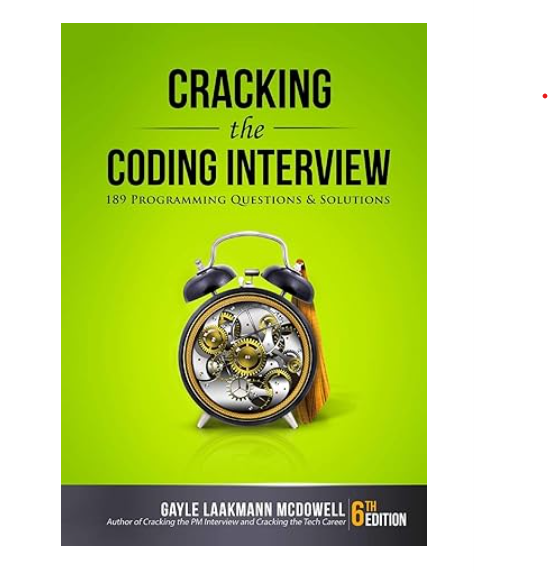
Java String split in Javascript With Example Code
Java String split() method is a useful method to split a string into an array of substrings based on a delimiter. However, if you are working with JavaScript, you might wonder how to achieve the same functionality. In this blog post, we will discuss how to Java String split in JavaScript.
To split a string in JavaScript, you can use the split() method of the String object. This method takes a delimiter as an argument and returns an array of substrings. Here is an example:
const str = "Hello World";
const arr = str.split(" ");
console.log(arr); // Output: ["Hello", "World"]
In this example, we have used the split() method to split the string “Hello World” into an array of two substrings “Hello” and “World” based on the space delimiter.
You can also use a regular expression as a delimiter. For example:
const str = "apple,banana,orange";
const arr = str.split(/,/);
console.log(arr); // Output: ["apple", "banana", "orange"]
In this example, we have used a regular expression /,/ as a delimiter to split the string “apple,banana,orange” into an array of three substrings “apple”, “banana”, and “orange”.
If you want to limit the number of substrings in the resulting array, you can pass a second argument to the split() method. For example:
const str = "apple,banana,orange";
const arr = str.split(",", 2);
console.log(arr); // Output: ["apple", "banana"]
In this example, we have used the split() method to split the string “apple,banana,orange” into an array of two substrings “apple” and “banana” based on the comma delimiter and limited the resulting array to two substrings.
In conclusion, splitting a string in JavaScript is easy using the split() method of the String object. You can use a delimiter or a regular expression as an argument and limit the number of substrings in the resulting array.
Equivalent of Java String split in Javascript
In conclusion, the equivalent function of Java’s String split() in JavaScript is the split() method. This method allows you to split a string into an array of substrings based on a specified separator. It is a powerful tool that can be used to manipulate and extract data from strings in JavaScript. By understanding the syntax and functionality of the split() method, you can easily split strings and perform various operations on the resulting array. Whether you are a beginner or an experienced JavaScript developer, the split() method is an essential tool to have in your arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |