The Java String split function is a method that allows a string to be split into an array of substrings based on a specified delimiter. The split function takes a regular expression as its argument, which is used to identify the delimiter. The resulting array contains all the substrings that were separated by the delimiter. The split function can be useful for parsing text data or breaking down a string into smaller components. It is commonly used in Java programming for data manipulation and processing. Keep reading below to learn how to Java String split in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
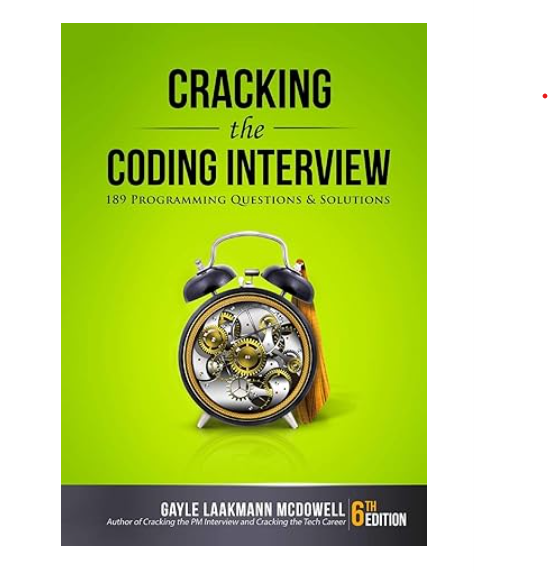
Java String split in Rust With Example Code
Java developers who are transitioning to Rust may find themselves wondering how to perform certain tasks in the new language. One such task is splitting a string, which is a common operation in many programs. In this blog post, we will explore how to split a string in Rust using the same approach as Java’s String.split() method.
To split a string in Rust, we can use the split() method provided by the standard library’s String type. This method returns an iterator that yields the substrings between the delimiter(s) we specify. Here’s an example:
let s = "hello world";
let words: Vec<&str> = s.split(" ").collect();
In this example, we split the string “hello world” into words by specifying a space (” “) as the delimiter. The split() method returns an iterator, which we collect into a vector of string slices (&str) using the collect() method.
If we want to split a string using multiple delimiters, we can pass a closure to the split() method that determines when to split the string. For example, to split a string using both spaces and commas as delimiters, we can do the following:
let s = "hello, world";
let words: Vec<&str> = s.split(|c| c == ' ' || c == ',').collect();
In this example, we pass a closure that checks if the current character is either a space or a comma. If it is, the string is split at that point.
In conclusion, splitting a string in Rust is a straightforward process that can be accomplished using the split() method provided by the standard library’s String type. By using closures, we can split a string using multiple delimiters or even more complex conditions.
Equivalent of Java String split in Rust
In conclusion, Rust provides a powerful and efficient way to split strings using the `split` function. This function is similar to the Java String `split` function, but with some differences in syntax and behavior. Rust’s `split` function allows for flexible and customizable splitting of strings based on a delimiter or a pattern. It also returns an iterator that can be used to iterate over the resulting substrings. Overall, Rust’s `split` function is a great tool for developers who need to manipulate strings in their Rust programs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |