The Java String split function is a method that allows a string to be split into an array of substrings based on a specified delimiter. The split function takes a regular expression as its argument, which is used to identify the delimiter. The resulting array contains all the substrings that were separated by the delimiter. The split function can be useful for parsing text data or breaking down a string into smaller components. It is commonly used in Java programming for data manipulation and processing. Keep reading below to learn how to Java String split in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
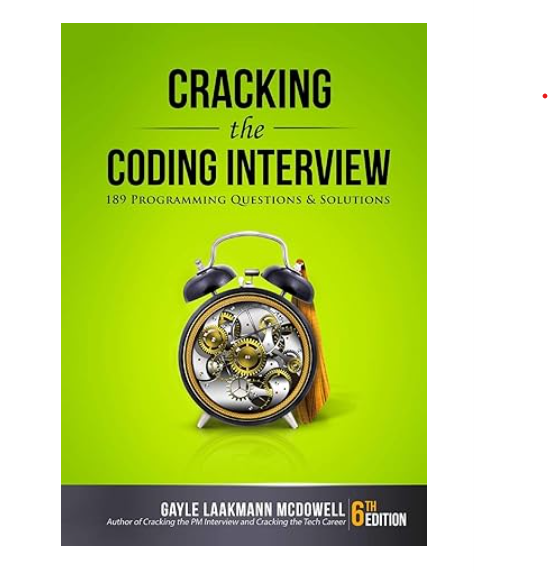
Java String split in TypeScript With Example Code
Java String split is a useful method that allows you to split a string into an array of substrings based on a specified delimiter. If you’re working with TypeScript, you may be wondering how to use this method in your code. In this blog post, we’ll walk through the steps to Java String split in TypeScript.
To use the Java String split method in TypeScript, you first need to import the String class from the java.lang package. You can do this using the following code:
import { String } from 'java.lang';
Once you’ve imported the String class, you can use the split method to split a string into an array of substrings. The split method takes a delimiter as its argument and returns an array of substrings. Here’s an example:
const str = 'Hello,World';
const arr = String(str).split(',');
console.log(arr); // Output: ['Hello', 'World']
In this example, we’re splitting the string ‘Hello,World’ using the comma delimiter. The split method returns an array containing the substrings ‘Hello’ and ‘World’, which we then log to the console.
It’s important to note that the split method returns an array of strings, so if you need to perform any operations on the substrings, you’ll need to convert them to the appropriate data type.
In conclusion, Java String split is a useful method that can be used in TypeScript by importing the String class from the java.lang package. By following the steps outlined in this blog post, you can easily split a string into an array of substrings based on a specified delimiter.
Equivalent of Java String split in TypeScript
In conclusion, TypeScript provides a powerful and efficient way to split strings using the `split()` function. This function works in a similar way to the equivalent Java String split function, allowing developers to easily split strings into arrays based on a specified delimiter. With TypeScript’s strong typing and object-oriented features, developers can write cleaner and more maintainable code when working with strings. Whether you’re working on a small project or a large-scale application, TypeScript’s `split()` function is a valuable tool to have in your toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |