The Java String startsWith function is a method that is used to check whether a given string starts with a specified prefix or not. It takes a single argument, which is the prefix to be checked, and returns a boolean value indicating whether the string starts with the prefix or not. The function is case-sensitive, meaning that it will return false if the prefix is not in the same case as the beginning of the string. This function is commonly used in string manipulation and searching operations, such as checking if a URL starts with “http://” or if a file name starts with a certain prefix. Keep reading below to learn how to Java String startsWith in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
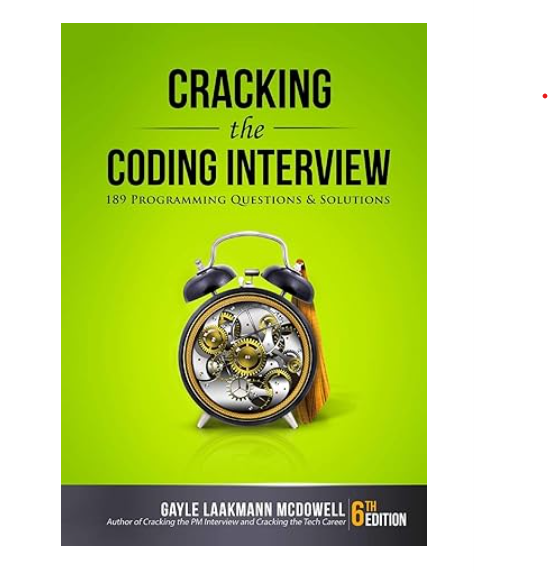
Java String startsWith in C++ With Example Code
Java has a built-in method called startsWith()
that allows you to check if a string starts with a specific prefix. This can be very useful when working with strings in Java. However, if you are working with C++, you may be wondering if there is a similar method available.
Fortunately, C++ does have a way to check if a string starts with a specific prefix. The method is called std::string::find()
. This method searches for a specific substring within a string and returns the position of the first occurrence of that substring. If the substring is found at the beginning of the string, the position returned will be 0.
To use std::string::find()
to check if a string starts with a specific prefix, you can simply search for the prefix and check if the position returned is 0. Here is an example:
#include <string>
#include <iostream>
int main() {
std::string str = "Hello, world!";
if (str.find("Hello") == 0) {
std::cout << "String starts with 'Hello'" << std::endl;
} else {
std::cout << "String does not start with 'Hello'" << std::endl;
}
return 0;
}
In this example, we create a string called str
and then use std::string::find()
to search for the prefix “Hello”. We then check if the position returned is 0 and print out a message accordingly.
So, while C++ does not have a built-in method called startsWith()
like Java does, you can still easily check if a string starts with a specific prefix using std::string::find()
.
Equivalent of Java String startsWith in C++
In conclusion, the equivalent function to Java’s String startsWith() in C++ is the substr() function. This function allows us to extract a substring from a given string and compare it with another string to check if it starts with that substring. While the syntax and implementation may differ between the two languages, the functionality remains the same. By understanding the similarities and differences between these two functions, developers can easily transition between Java and C++ and write efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |