The Java String startsWith function is a method that is used to check whether a given string starts with a specified prefix or not. It takes a single argument, which is the prefix to be checked, and returns a boolean value indicating whether the string starts with the prefix or not. The function is case-sensitive, meaning that it will return false if the prefix is not in the same case as the beginning of the string. This function is commonly used in string manipulation and searching operations, such as checking if a URL starts with “http://” or if a file name starts with a certain prefix. Keep reading below to learn how to Java String startsWith in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
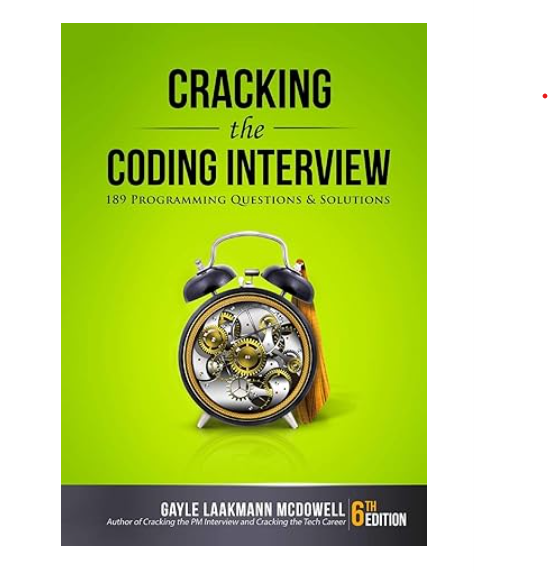
Java String startsWith in Kotlin With Example Code
Java String’s `startsWith()` method is a useful tool for checking if a string starts with a specific prefix. In Kotlin, this method can be used in a similar way.
To use `startsWith()` in Kotlin, simply call the method on a string and pass in the prefix you want to check for. The method will return a boolean value indicating whether or not the string starts with the specified prefix.
Here’s an example of how to use `startsWith()` in Kotlin:
val str = "Hello, world!"
val prefix = "Hello"
if (str.startsWith(prefix)) {
println("The string starts with $prefix")
} else {
println("The string does not start with $prefix")
}
In this example, we create a string `str` and a prefix `prefix`. We then use `startsWith()` to check if `str` starts with `prefix`. If it does, we print a message indicating that the string starts with the prefix. If it doesn’t, we print a message indicating that the string does not start with the prefix.
Overall, `startsWith()` is a simple and useful method for checking if a string starts with a specific prefix in Kotlin.
Equivalent of Java String startsWith in Kotlin
In conclusion, the Kotlin programming language provides a more concise and efficient way of working with strings compared to Java. The equivalent Kotlin function for Java’s startsWith() method is the startsWith() function, which is more intuitive and easier to use. With this function, developers can easily check if a string starts with a specific prefix without having to write lengthy code. Additionally, Kotlin’s string interpolation feature allows for more readable and maintainable code when working with strings. Overall, Kotlin’s string handling capabilities make it a great choice for developers looking to build robust and efficient applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |