The Java String startsWith function is a method that is used to check whether a given string starts with a specified prefix or not. It takes a single argument, which is the prefix to be checked, and returns a boolean value indicating whether the string starts with the prefix or not. The function is case-sensitive, meaning that it will return false if the prefix is not in the same case as the beginning of the string. This function is commonly used in string manipulation and searching operations, such as checking if a URL starts with “http://” or if a file name starts with a certain prefix. Keep reading below to learn how to Java String startsWith in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
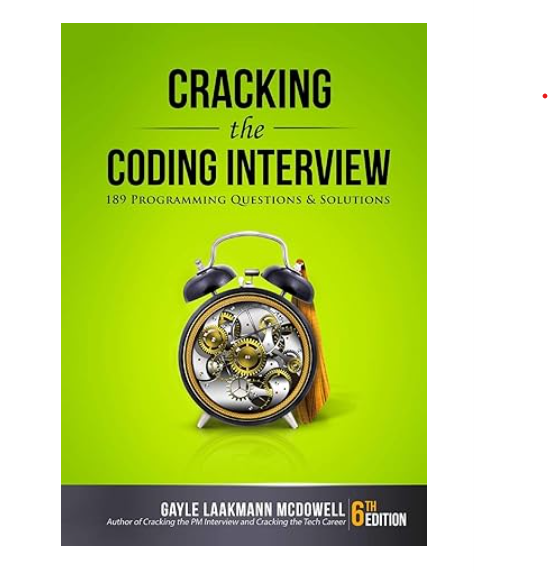
Java String startsWith in TypeScript With Example Code
Java’s `startsWith` method is a useful tool for checking if a string starts with a certain prefix. If you’re working with TypeScript, you might be wondering how to achieve the same functionality. Fortunately, TypeScript has a similar method that you can use: `startsWith`.
To use `startsWith` in TypeScript, you can simply call the method on a string and pass in the prefix you want to check for. Here’s an example:
const myString = "Hello, world!";
const prefix = "Hello";
if (myString.startsWith(prefix)) {
console.log("The string starts with the prefix!");
} else {
console.log("The string does not start with the prefix.");
}
In this example, we’re checking if `myString` starts with the prefix “Hello”. If it does, we log a message saying so. If it doesn’t, we log a different message.
It’s worth noting that `startsWith` is case-sensitive, so if you want to check for a prefix regardless of case, you’ll need to convert both the string and the prefix to lowercase (or uppercase) before calling the method.
Overall, `startsWith` is a simple and effective way to check if a string starts with a certain prefix in TypeScript.
Equivalent of Java String startsWith in TypeScript
In conclusion, TypeScript provides a powerful and efficient way to work with strings in your code. The equivalent Java String startsWith function in TypeScript is the startsWith() method, which allows you to check if a string starts with a specific substring. This method is easy to use and provides a reliable way to perform string comparisons in your TypeScript code. By leveraging the power of TypeScript’s string manipulation capabilities, you can write cleaner, more efficient code that is easier to maintain and debug. Whether you are a seasoned developer or just starting out with TypeScript, the startsWith() method is a valuable tool to have in your toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |