The Java String subSequence function is used to extract a portion of a string and return it as a new string. It takes two parameters: the starting index and the ending index (exclusive) of the substring to be extracted. The function creates a new string that contains the characters from the original string starting at the specified index and ending at the specified index (exclusive). The original string is not modified. The subSequence function is useful when you need to extract a portion of a string for further processing or manipulation. Keep reading below to learn how to Java String subSequence in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
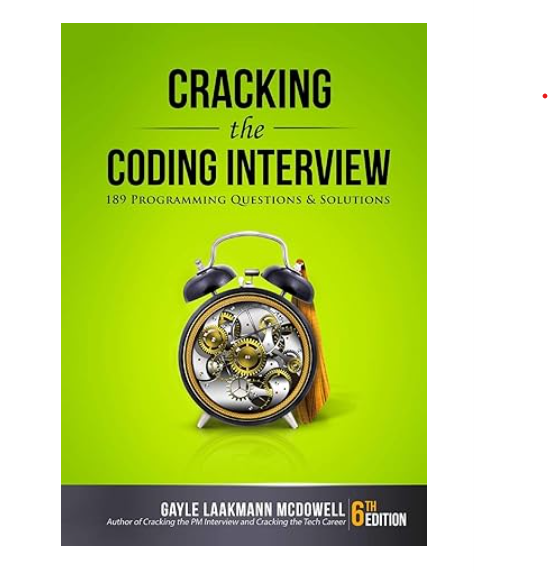
Java String subSequence in C++ With Example Code
Java’s String class has a method called subSequence() that returns a new character sequence that is a subsequence of the original sequence. This method can be useful when you want to extract a portion of a string. In C++, there is no built-in method for subsequence, but you can easily create your own.
To create a subsequence in C++, you can use the substr() method of the string class. This method takes two arguments: the starting index and the length of the subsequence. Here’s an example:
#include
#include
using namespace std;
int main() {
string str = "Hello, world!";
string sub = str.substr(7, 5);
cout << sub << endl;
return 0;
}
In this example, we create a string called "str" with the value "Hello, world!". We then create a new string called "sub" by calling the substr() method on "str" with the arguments 7 and 5. This means that we want to start at index 7 (which is the letter "w") and extract 5 characters (which gives us "world").
When we run this program, it will output "world" to the console.
So, while C++ doesn't have a built-in subSequence() method like Java, you can easily create your own using the substr() method of the string class.
Equivalent of Java String subSequence in C++
In conclusion, the equivalent function to Java's String subSequence in C++ is the substr() function. This function allows you to extract a portion of a string based on a starting index and a length. It is a powerful tool that can be used in a variety of applications, from manipulating text to parsing data. By understanding the similarities and differences between these two functions, developers can write more efficient and effective code in both Java and C++. Whether you are a seasoned programmer or just starting out, the substr() function is a valuable tool to have in your programming arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |