The Java String subSequence function is used to extract a portion of a string and return it as a new string. It takes two parameters: the starting index and the ending index (exclusive) of the substring to be extracted. The function returns a CharSequence object, which can be cast to a String if needed. The subSequence function does not modify the original string, but instead creates a new string that contains the specified portion of the original string. This function is useful when you need to work with a specific part of a larger string, such as extracting a username from an email address. Keep reading below to learn how to Java String subSequence in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
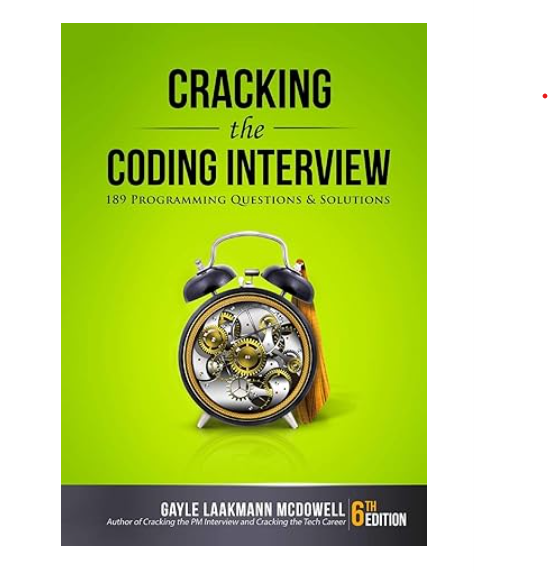
Java String subSequence in Kotlin With Example Code
Java String subSequence is a method that allows you to extract a portion of a string based on the starting and ending index. In Kotlin, you can use the same method by calling the `subSequence` function on a string.
To use the `subSequence` function, you need to provide the starting and ending index of the substring you want to extract. The starting index is inclusive, while the ending index is exclusive. This means that the character at the ending index is not included in the substring.
Here’s an example code snippet that demonstrates how to use the `subSequence` function in Kotlin:
val str = "Hello, World!"
val sub = str.subSequence(0, 5)
println(sub) // Output: Hello
In this example, we create a string `str` that contains the text “Hello, World!”. We then call the `subSequence` function on the string and provide the starting index of 0 and the ending index of 5. This extracts the substring “Hello” from the original string.
You can also use the `subSequence` function with variables that hold string values. Here’s an example:
val name = "John Doe"
val subName = name.subSequence(0, 4)
println(subName) // Output: John
In this example, we create a variable `name` that holds the string value “John Doe”. We then call the `subSequence` function on the variable and provide the starting index of 0 and the ending index of 4. This extracts the substring “John” from the original string.
In conclusion, the `subSequence` function in Kotlin allows you to extract substrings from a string based on the starting and ending index. It’s a useful method that can come in handy when working with strings in Kotlin.
Equivalent of Java String subSequence in Kotlin
In conclusion, the Kotlin programming language provides a convenient and efficient way to manipulate strings using the subSequence function. This function is equivalent to the Java String subSequence function and allows developers to extract a portion of a string based on a specified range of indices. With its simple syntax and powerful capabilities, the subSequence function in Kotlin is a valuable tool for any developer working with strings. Whether you are a beginner or an experienced programmer, mastering this function will help you write cleaner, more efficient code and make your applications more robust and reliable. So, if you’re looking to take your Kotlin programming skills to the next level, be sure to explore the subSequence function and all the other powerful string manipulation tools that Kotlin has to offer.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |