The Java String subSequence function is used to extract a portion of a string and return it as a new string. It takes two parameters: the starting index and the ending index (exclusive) of the substring to be extracted. The function creates a new string that contains the characters from the original string starting at the specified index and ending at the specified index (exclusive). The original string is not modified. The subSequence function is useful when you need to extract a portion of a string for further processing or manipulation. Keep reading below to learn how to Java String subSequence in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
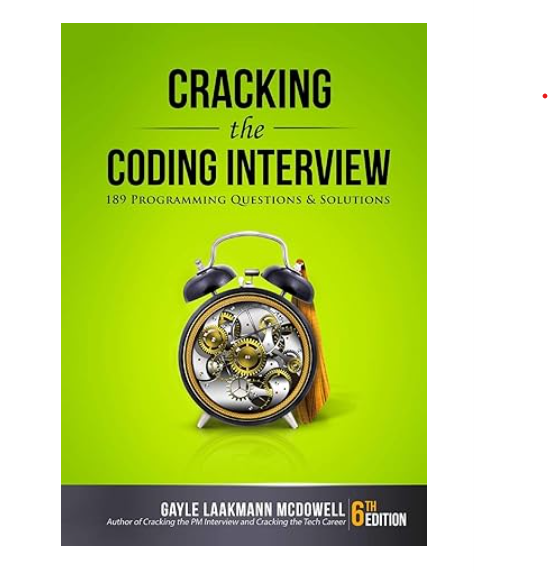
Java String subSequence in TypeScript With Example Code
Java String subSequence is a useful method that allows you to extract a portion of a string based on its starting and ending indices. If you’re working with TypeScript and need to perform a similar operation, you can use the substring method. In this blog post, we’ll explore how to use the substring method in TypeScript.
To use the substring method, you first need to create a string variable. Let’s say you have a string variable called “myString” that contains the value “Hello, world!”. To extract a portion of this string, you can use the substring method as follows:
let mySubstring = myString.substring(startIndex, endIndex);
In this example, “startIndex” is the index of the first character you want to include in the substring, and “endIndex” is the index of the first character you want to exclude from the substring. For example, if you want to extract the word “world” from the string, you can use the following code:
let mySubstring = myString.substring(7, 12);
This will create a new string variable called “mySubstring” that contains the value “world”.
It’s important to note that the substring method is zero-indexed, which means that the first character in the string has an index of 0, the second character has an index of 1, and so on. Additionally, if you omit the “endIndex” parameter, the substring method will extract all characters from the “startIndex” to the end of the string.
In conclusion, the substring method in TypeScript is a powerful tool that allows you to extract portions of a string based on their indices. By using this method, you can easily manipulate strings in your TypeScript code.
Equivalent of Java String subSequence in TypeScript
In conclusion, TypeScript provides a powerful and efficient way to manipulate strings using the subSequence function. This function is equivalent to the Java String subSequence function and allows developers to extract a portion of a string based on a specified start and end index. With TypeScript’s strong typing and object-oriented features, developers can easily work with strings and perform various operations on them. Whether you are working on a small project or a large-scale application, TypeScript’s subSequence function can help you efficiently manipulate strings and achieve your desired results. So, if you are looking for a reliable and efficient way to work with strings in TypeScript, the subSequence function is definitely worth exploring.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |