The Java String substring function is used to extract a portion of a string. It takes two parameters: the starting index and the ending index of the substring. The starting index is inclusive, meaning the character at that index is included in the substring, while the ending index is exclusive, meaning the character at that index is not included in the substring. If only the starting index is provided, the substring will include all characters from that index to the end of the string. The substring function returns a new string that contains the extracted portion of the original string. Keep reading below to learn how to Java String substring in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
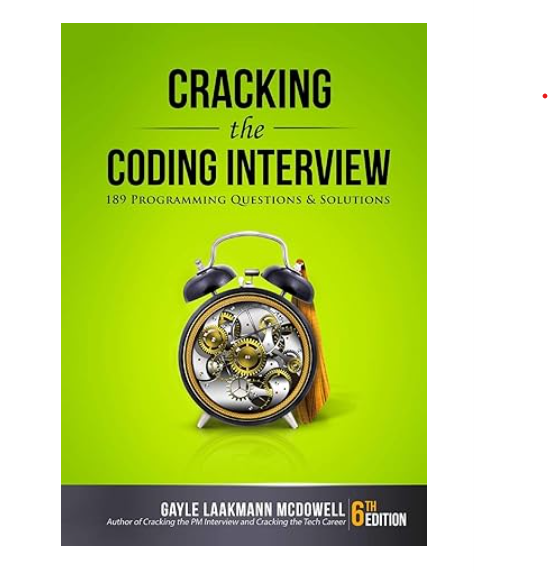
Java String substring in C# With Example Code
Java String substring is a commonly used method in Java programming. However, if you are working with C#, you may be wondering how to achieve the same functionality. In this blog post, we will explore how to Java String substring in C#.
To Java String substring in C#, you can use the Substring method. This method is available in the System namespace and can be used to extract a substring from a string.
The Substring method takes two parameters: the starting index and the length of the substring. The starting index is the position of the first character in the substring, and the length is the number of characters to include in the substring.
Here is an example of how to use the Substring method in C#:
string str = "Hello World";
string substr = str.Substring(6, 5);
Console.WriteLine(substr); // Output: World
In this example, we have a string “Hello World”. We then use the Substring method to extract a substring starting at index 6 (which is the letter “W”) and including 5 characters. The resulting substring is “World”, which is then printed to the console.
It is important to note that the Substring method is zero-indexed, meaning that the first character in a string has an index of 0. Additionally, if you do not specify a length parameter, the Substring method will return all characters from the starting index to the end of the string.
In conclusion, Java String substring functionality can be achieved in C# using the Substring method. By specifying the starting index and length of the substring, you can extract a portion of a string in C#.
Equivalent of Java String substring in C#
In conclusion, the Java String substring function and its equivalent in C# are very similar in terms of functionality and syntax. Both functions allow developers to extract a portion of a string based on a specified starting index and length. However, there are some minor differences in the way these functions are implemented in each language. For example, the C# substring function uses a zero-based index for the starting position, while the Java substring function uses a one-based index. Additionally, the C# substring function throws an exception if the specified length exceeds the length of the string, while the Java substring function simply returns the remaining characters of the string. Despite these differences, both functions are powerful tools for manipulating strings in their respective languages, and can be used to accomplish a wide range of tasks in any C# or Java project.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |