The Java String substring function is used to extract a portion of a string. It takes two parameters: the starting index and the ending index of the substring. The starting index is inclusive, meaning the character at that index is included in the substring, while the ending index is exclusive, meaning the character at that index is not included in the substring. If only the starting index is provided, the substring will include all characters from that index to the end of the string. The substring function returns a new string that contains the extracted portion of the original string. Keep reading below to learn how to Java String substring in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
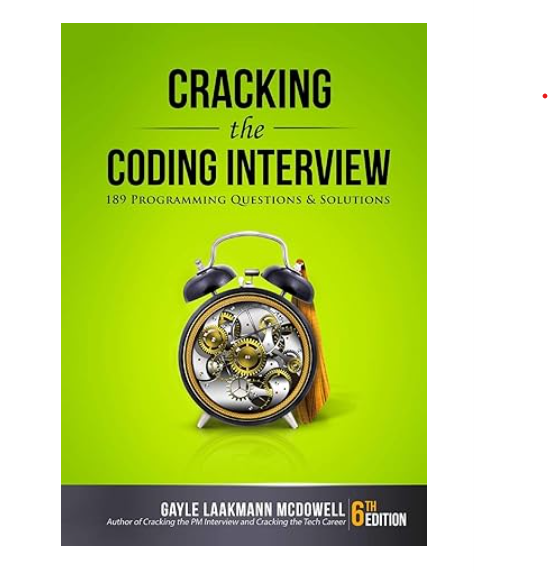
Java String substring in Python With Example Code
Java and Python are two of the most popular programming languages in the world. While they have many similarities, there are also some differences between them. One of these differences is how they handle string manipulation. In Java, there is a built-in method called substring() that allows you to extract a portion of a string. In Python, there is no equivalent method, but there are several ways to achieve the same result.
To Java developers who are new to Python, this can be a bit confusing. In this blog post, we will explore how to Java String substring in Python.
The first method to achieve substring functionality in Python is by using slicing. Slicing allows you to extract a portion of a string by specifying the start and end indices. Here is an example:
string = "Hello, World!"
substring = string[0:5]
print(substring)
This will output “Hello”, which is the first five characters of the string.
Another way to achieve substring functionality in Python is by using the split() method. This method splits a string into a list of substrings based on a delimiter. Here is an example:
string = "Hello, World!"
substring = string.split(",")[0]
print(substring)
This will output “Hello”, which is the first substring before the comma.
A third way to achieve substring functionality in Python is by using regular expressions. Regular expressions are a powerful tool for pattern matching and string manipulation. Here is an example:
import re
string = "Hello, World!"
substring = re.search("Hello", string).group()
print(substring)
This will output “Hello”, which is the first occurrence of the word “Hello” in the string.
In conclusion, while there is no built-in method for substring in Python like there is in Java, there are several ways to achieve the same result. Slicing, splitting, and regular expressions are all powerful tools for string manipulation in Python.
Equivalent of Java String substring in Python
In conclusion, Python’s equivalent to Java’s String substring function is the string slicing feature. This feature allows us to extract a portion of a string by specifying the start and end indices. The syntax for string slicing in Python is simple and intuitive, making it easy for developers to use. Additionally, Python’s string slicing feature is more flexible than Java’s substring function, as it allows us to extract substrings from a string in multiple ways. Overall, Python’s string slicing feature is a powerful tool that can help developers manipulate strings with ease.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |