The Java String toCharArray function is a method that converts a string into an array of characters. It returns a new character array that contains the same sequence of characters as the original string. This function is useful when you need to manipulate individual characters in a string, such as sorting or searching for specific characters. The resulting character array can be used in various operations, such as concatenation or comparison with other character arrays. The toCharArray function is a built-in method in the Java String class and can be called on any string object. Keep reading below to learn how to Java String toCharArray in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
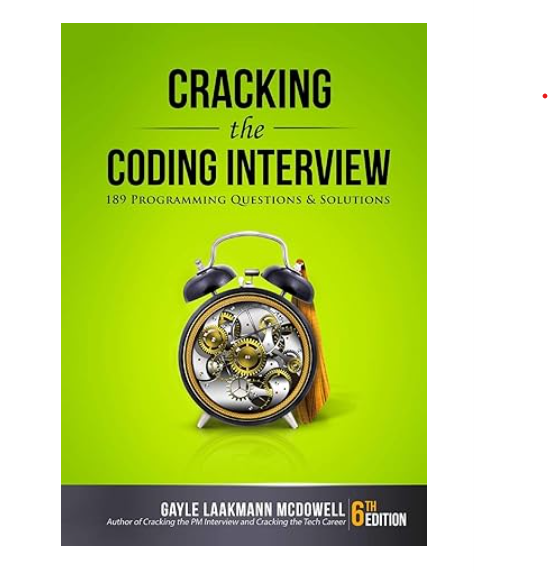
Java String toCharArray in C++ With Example Code
Converting a Java String to a char array in C++ can be done using the c_str()
function. This function returns a pointer to an array that contains a null-terminated sequence of characters representing the string.
Here is an example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World!";
const char* char_array = str.c_str();
cout << char_array << endl;
return 0;
}
In this example, we first create a string
object called str
that contains the string “Hello World!”. We then use the c_str()
function to convert the string to a const char pointer called char_array
. Finally, we print out the contents of char_array
using the cout
statement.
Equivalent of Java String toCharArray in C++
In conclusion, the equivalent function to Java’s String toCharArray() in C++ is the string’s member function c_str(). This function returns a pointer to an array that contains a null-terminated sequence of characters representing the string’s contents. This array can be accessed and manipulated just like any other C-style string. It is important to note that the returned pointer should not be modified, as it points to the internal representation of the string. Overall, the c_str() function provides a convenient way to convert a C++ string to a C-style string, making it easier to work with in certain situations.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |