The Java String toCharArray function is a built-in method that converts a string into an array of characters. It returns a new character array that contains the same sequence of characters as the original string. This function is useful when you need to manipulate individual characters in a string, such as sorting or searching for specific characters. The resulting character array can be used in various operations, such as concatenation or comparison with other character arrays. The toCharArray function takes no arguments and returns an array of characters. Keep reading below to learn how to Java String toCharArray in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
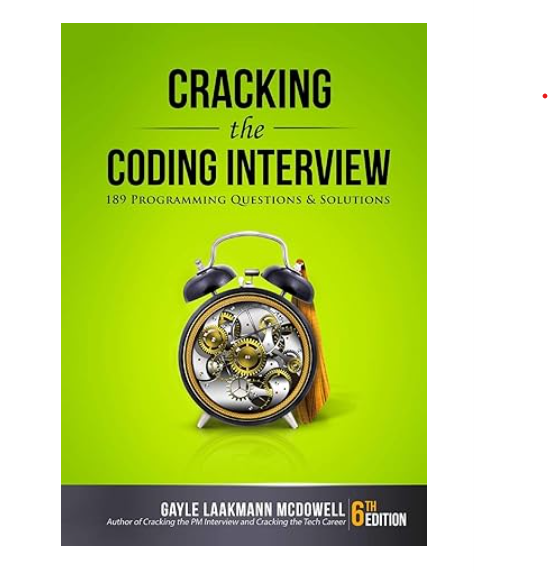
Java String toCharArray in Javascript With Example Code
Converting a Java String to a char array in JavaScript can be useful in a variety of situations. Fortunately, it’s a relatively simple process.
First, let’s take a look at the Java code for converting a String to a char array:
char[] charArray = str.toCharArray();
In JavaScript, we can achieve the same result using the split()
method. Here’s an example:
var str = "Hello, world!";
var charArray = str.split('');
In this example, we’re splitting the string into an array of characters using an empty string as the separator. This effectively splits the string at every character, resulting in an array of characters.
It’s worth noting that the resulting array will be an array of strings, not an array of characters. To convert each string element to a character, we can use the charAt()
method:
for (var i = 0; i < charArray.length; i++) {
charArray[i] = charArray[i].charAt(0);
}
In this example, we're iterating over each element in the array and replacing it with the first character of that element.
And that's it! With these simple steps, you can easily convert a Java String to a char array in JavaScript.
Equivalent of Java String toCharArray in Javascript
In conclusion, the equivalent function to Java's toCharArray() in JavaScript is the split() method. Both functions serve the same purpose of converting a string into an array of characters. However, the split() method in JavaScript has some additional features that make it more versatile than toCharArray() in Java. For instance, the split() method can split a string into an array of substrings based on a specified separator, while toCharArray() only splits a string into an array of characters. Therefore, if you are working with JavaScript, you can use the split() method to convert a string into an array of characters, and also split the string into substrings if needed.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |