The Java String toLowerCase() function is a built-in method that converts all the characters in a given string to lowercase. This function returns a new string with all the characters in lowercase. It is useful when we want to compare two strings without considering their case sensitivity. The toLowerCase() function does not modify the original string, but instead creates a new string with all the characters in lowercase. This function is a part of the String class in Java and can be called on any string object. Keep reading below to learn how to Java String toLowerCase in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
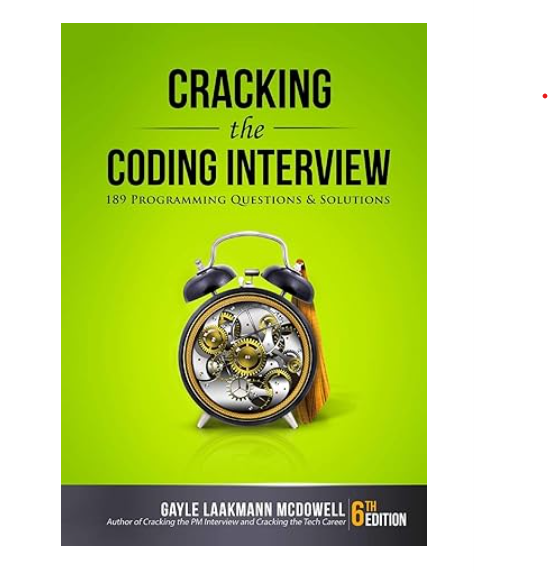
Java String toLowerCase in C# With Example Code
Converting a Java String to lowercase in C# is a common task when working with strings. Fortunately, C# provides a built-in method to accomplish this task.
The ToLower()
method is used to convert a string to lowercase in C#. This method returns a new string that is the lowercase version of the original string.
Here is an example of how to use the ToLower()
method:
string myString = "HELLO WORLD";
string lowerString = myString.ToLower();
Console.WriteLine(lowerString); // Output: hello world
In this example, the ToLower()
method is called on the myString
variable, which contains the string “HELLO WORLD”. The resulting string, “hello world”, is then stored in the lowerString
variable.
It is important to note that the ToLower()
method returns a new string and does not modify the original string. If you want to modify the original string, you can assign the result of the ToLower()
method back to the original variable:
string myString = "HELLO WORLD";
myString = myString.ToLower();
Console.WriteLine(myString); // Output: hello world
Using the ToLower()
method is a simple and effective way to convert a Java String to lowercase in C#.
Equivalent of Java String toLowerCase in C#
In conclusion, the equivalent Java String toLowerCase function in C# is the ToLower() method. This method is used to convert all the characters in a string to lowercase. It is a simple and efficient way to manipulate strings in C#. By using this method, developers can easily convert strings to lowercase without having to write complex code. Additionally, the ToLower() method is part of the .NET framework, which means it is readily available for use in C# applications. Overall, the ToLower() method is a valuable tool for developers who need to manipulate strings in C#.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |