The Java String toLowerCase() function is a built-in method that converts all the characters in a given string to lowercase. This function returns a new string with all the characters in lowercase. It is useful when we want to compare two strings without considering their case sensitivity. The toLowerCase() function does not modify the original string, but instead creates a new string with all the characters in lowercase. This function is a part of the String class in Java and can be called on any string object. Keep reading below to learn how to Java String toLowerCase in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
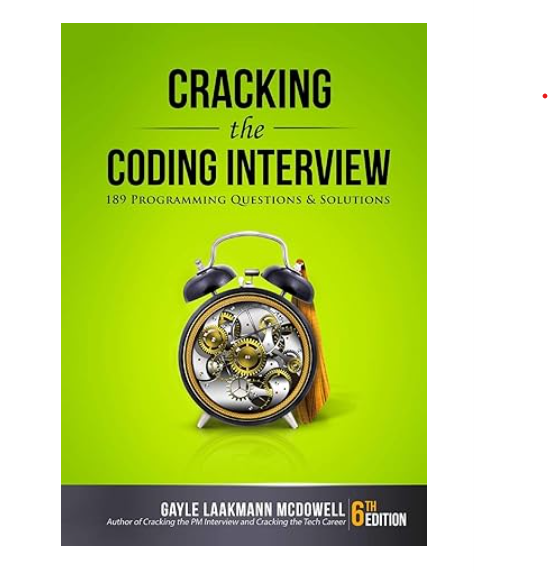
Java String toLowerCase in Rust With Example Code
Converting a Java String to lowercase in Rust is a common task when working with strings. In Rust, you can use the `to_lowercase()` method to convert a string to lowercase.
Here’s an example of how to use the `to_lowercase()` method:
let my_string = "HELLO, WORLD!";
let lowercase_string = my_string.to_lowercase();
println!("{}", lowercase_string);
In this example, we create a string called `my_string` that contains the text “HELLO, WORLD!”. We then call the `to_lowercase()` method on `my_string` to convert it to lowercase. Finally, we print the lowercase string to the console.
It’s important to note that the `to_lowercase()` method returns a new string that is the lowercase version of the original string. The original string is not modified.
Here’s another example that shows how to convert a user input string to lowercase:
use std::io;
fn main() {
println!("Enter a string:");
let mut input = String::new();
io::stdin().read_line(&mut input).expect("Failed to read line");
let lowercase_input = input.to_lowercase();
println!("Lowercase string: {}", lowercase_input);
}
In this example, we use the `std::io` module to read a string input from the user. We then call the `to_lowercase()` method on the input string to convert it to lowercase. Finally, we print the lowercase string to the console.
In conclusion, converting a Java String to lowercase in Rust is a simple task that can be accomplished using the `to_lowercase()` method. This method returns a new string that is the lowercase version of the original string.
Equivalent of Java String toLowerCase in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to convert strings to lowercase using the `to_lowercase()` function. This function is equivalent to the Java `toLowerCase()` function and can be used to convert any string to lowercase, regardless of its original case. With Rust’s focus on performance and safety, developers can be confident that their code will run smoothly and reliably, even when dealing with large amounts of data. Whether you’re a seasoned Rust developer or just getting started, the `to_lowercase()` function is a valuable tool to have in your arsenal for working with strings.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |