The Java String toLowerCase() function is a built-in method that converts all the characters in a given string to lowercase. This function returns a new string with all the characters in lowercase. It is useful when we want to compare two strings without considering their case sensitivity. The toLowerCase() function does not modify the original string, but instead creates a new string with all the characters in lowercase. This function is easy to use and can be applied to any string variable or string literal in Java. Keep reading below to learn how to Java String toLowerCase in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
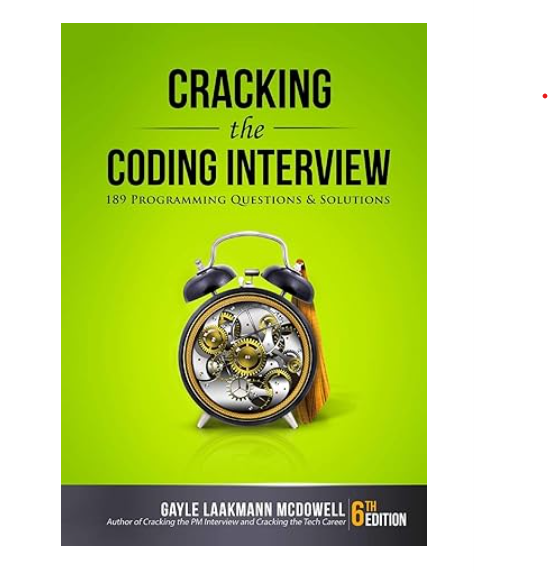
Java String toLowerCase in TypeScript With Example Code
To convert a Java String to lowercase in TypeScript, we can use the `toLowerCase()` method. This method returns a new string with all the characters in lowercase.
Here’s an example:
const myString = "HELLO WORLD";
const lowerCaseString = myString.toLowerCase();
console.log(lowerCaseString); // Output: "hello world"
In the example above, we first declare a string variable `myString` with the value “HELLO WORLD”. We then call the `toLowerCase()` method on this string and assign the result to a new variable `lowerCaseString`. Finally, we log the value of `lowerCaseString` to the console, which outputs “hello world”.
It’s important to note that the `toLowerCase()` method does not modify the original string. Instead, it returns a new string with all the characters in lowercase. If you want to modify the original string, you can assign the result of `toLowerCase()` back to the original variable.
In summary, converting a Java String to lowercase in TypeScript is as simple as calling the `toLowerCase()` method on the string.
Equivalent of Java String toLowerCase in TypeScript
In conclusion, the TypeScript language provides a powerful and convenient way to convert strings to lowercase using the toLowerCase() function. This function works in a similar way to the equivalent Java String toLowerCase() function, allowing developers to easily manipulate strings in their TypeScript code. Whether you are working on a small project or a large-scale application, the toLowerCase() function can help you to streamline your code and improve the readability of your codebase. So, if you are looking for a simple and effective way to convert strings to lowercase in TypeScript, the toLowerCase() function is definitely worth considering.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |