The Java String trim function is a built-in method that removes any leading and trailing white spaces from a given string. It returns a new string with the white spaces removed. The trim function is useful when dealing with user input or when parsing data from external sources, as it ensures that any extra spaces are removed before processing the data. The trim function can be called on any string object and does not modify the original string. Keep reading below to learn how to Java String trim in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
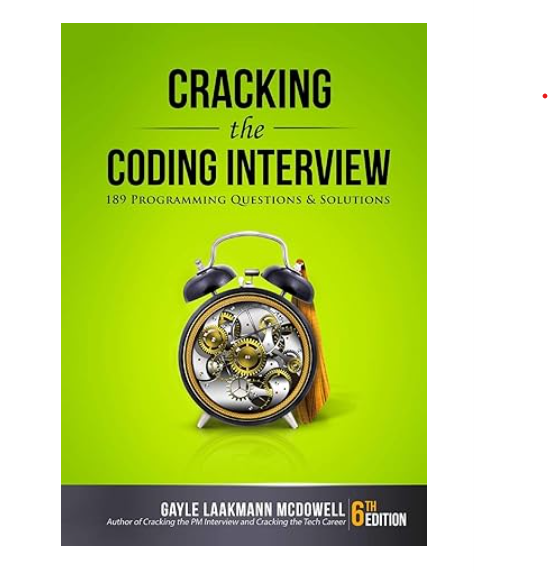
Java String trim in Python With Example Code
Java and Python are two of the most popular programming languages in the world. While they have many similarities, there are also some differences between them. One of these differences is how they handle string manipulation. In Java, there is a built-in method called “trim” that removes whitespace from the beginning and end of a string. In Python, there is no built-in method for this, but there are several ways to achieve the same result.
One way to trim a string in Python is to use the “strip” method. This method removes whitespace from the beginning and end of a string, just like the “trim” method in Java. Here is an example:
string = " hello world "
trimmed_string = string.strip()
print(trimmed_string)
This will output “hello world” without any leading or trailing whitespace.
Another way to trim a string in Python is to use regular expressions. Regular expressions are a powerful tool for manipulating strings, and they can be used to remove whitespace from the beginning and end of a string. Here is an example:
import re
string = " hello world "
trimmed_string = re.sub(r'^\s+|\s+$', '', string)
print(trimmed_string)
This will also output “hello world” without any leading or trailing whitespace.
In conclusion, while Java and Python handle string manipulation differently, there are ways to achieve the same result in both languages. By using the “strip” method or regular expressions, you can trim whitespace from the beginning and end of a string in Python, just like you would with the “trim” method in Java.
Equivalent of Java String trim in Python
In conclusion, the equivalent function of Java’s String trim() in Python is the strip() function. Both functions remove any leading or trailing whitespace from a string. While the syntax and implementation may differ slightly between the two languages, the end result is the same. It is important to note that the strip() function in Python can also remove other specified characters from a string, making it a versatile tool for string manipulation. Overall, understanding the equivalent functions in different programming languages can help developers work more efficiently and effectively across different platforms.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |