The Java String valueOf function is a method that converts different types of data into a string representation. It can be used to convert primitive data types such as int, float, double, and boolean, as well as objects such as arrays and characters, into a string. The valueOf function returns a string object that represents the specified value. It is commonly used in Java programming to convert data types for display or manipulation purposes. The syntax for the valueOf function is: String.valueOf(data). Keep reading below to learn how to Java String valueOf in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
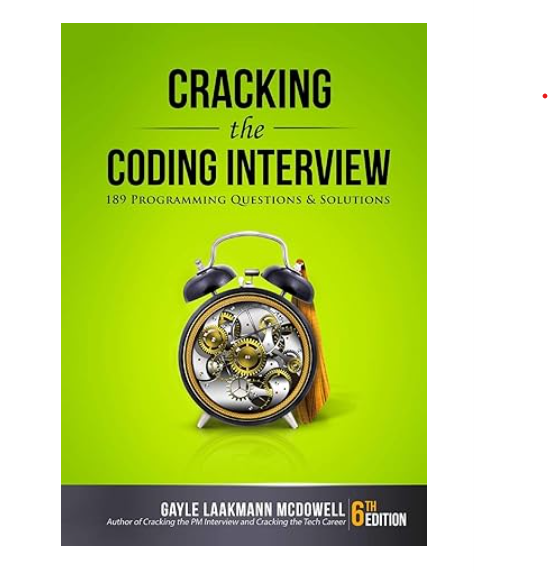
Java String valueOf in Bash With Example Code
Java’s String class is a powerful tool for manipulating strings in Java. However, what if you need to use a Java String method in a Bash script? One such method is `valueOf()`, which converts different types of data into a string. In this blog post, we will explore how to use Java’s `valueOf()` method in Bash.
First, we need to have Java installed on our system. We can check if Java is installed by running the following command in the terminal:
java -version
If Java is not installed, we can install it using the following command:
sudo apt-get install default-jre
Once we have Java installed, we can use the `java` command to execute Java code from within our Bash script. To use the `valueOf()` method, we need to create a Java class that contains the method. Here is an example Java class:
public class StringConverter {
public static String convertToString(Object obj) {
return String.valueOf(obj);
}
}
This class contains a static method called `convertToString()` that takes an `Object` as a parameter and returns a `String` representation of that object using the `valueOf()` method.
To use this class in our Bash script, we need to compile it using the `javac` command:
javac StringConverter.java
This will create a `StringConverter.class` file in the same directory as the `StringConverter.java` file.
Now, we can use the `java` command to execute the `convertToString()` method from within our Bash script. Here is an example Bash script that uses the `convertToString()` method to convert an integer to a string:
#!/bin/bash
# Compile the Java class
javac StringConverter.java
# Convert an integer to a string
result=$(java StringConverter 42)
# Print the result
echo $result
In this script, we first compile the `StringConverter.java` file using the `javac` command. Then, we use the `java` command to execute the `convertToString()` method with an integer value of `42`. Finally, we print the result, which should be the string `”42″`.
In conclusion, using Java’s `valueOf()` method in Bash is possible by creating a Java class that contains the method and using the `java` command to execute it from within our Bash script.
Equivalent of Java String valueOf in Bash
In conclusion, the Bash equivalent of the Java String valueOf function is the printf command. This command allows you to format and print variables as strings, making it a powerful tool for manipulating and displaying data in Bash scripts. By using the printf command, you can easily convert variables of different data types into strings, just like the String valueOf function in Java. Whether you’re working on a small Bash script or a larger project, understanding the printf command can help you write more efficient and effective code. So, if you’re looking for a way to convert variables to strings in Bash, give the printf command a try!
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |