The Java String valueOf function is a method that converts different types of data into a string representation. It can be used to convert primitive data types such as int, float, double, and boolean, as well as objects such as arrays and characters, into a string. The valueOf function returns a string object that represents the specified value. This method is useful when you need to concatenate different types of data into a single string or when you need to convert a non-string value into a string for display or manipulation purposes. Keep reading below to learn how to Java String valueOf in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
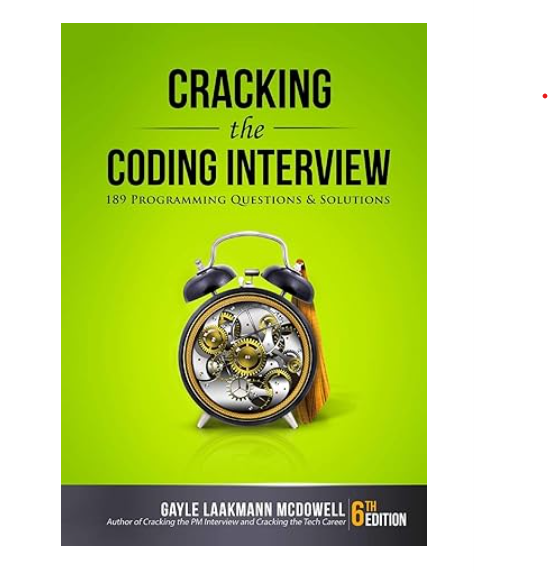
Java String valueOf in C++ With Example Code
Java’s String class has a method called `valueOf()` that converts different types of data into a string. This method is very useful when you need to convert data into a string for display or manipulation purposes. C++ does not have a built-in method for this, but it is possible to create a similar function.
To create a function that behaves like Java’s `valueOf()`, you can use C++’s `stringstream` class. This class allows you to convert different types of data into a string. Here is an example of how to use `stringstream` to create a `valueOf()` function in C++:
#include
#include
template
std::string valueOf(T value) {
std::stringstream ss;
ss << value;
return ss.str();
}
In this example, we create a template function called `valueOf()` that takes a value of any type and returns a string. Inside the function, we create a `stringstream` object and use the `<<` operator to insert the value into the stream. Finally, we use the `str()` method to convert the stream into a string and return it.
Here is an example of how to use the `valueOf()` function:
int main() {
int num = 42;
std::string str = valueOf(num);
std::cout << str << std::endl;
return 0;
}
In this example, we create an integer variable called `num` and set it to 42. We then call the `valueOf()` function with `num` as the argument and store the result in a string variable called `str`. Finally, we print out the value of `str` using `cout`.
With this `valueOf()` function, you can easily convert different types of data into strings in C++, just like you can in Java with the `valueOf()` method.
Equivalent of Java String valueOf in C++
In conclusion, the C++ equivalent of the Java String valueOf function is the std::to_string() function. This function allows C++ programmers to convert various data types into string format, just like the Java String valueOf function. It is a simple and efficient way to convert numerical values into strings, making it easier to manipulate and display data in C++ programs. By using the std::to_string() function, C++ programmers can easily convert integers, floats, and doubles into strings without having to write complex code. Overall, the std::to_string() function is a valuable tool for C++ programmers who need to convert numerical values into strings.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |