The Java String valueOf function is a method that converts different types of data into a string representation. It can be used to convert primitive data types such as int, float, double, and boolean, as well as objects such as arrays and characters, into a string. The valueOf function returns a string object that represents the specified value. This method is useful when you need to concatenate different types of data into a single string or when you need to convert a non-string value into a string for display or manipulation purposes. Keep reading below to learn how to Java String valueOf in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
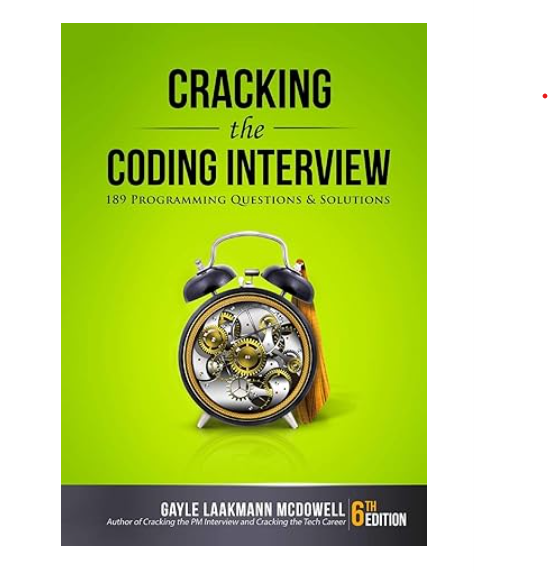
Java String valueOf in Go With Example Code
Java’s `valueOf()` method is a useful tool for converting data types into strings. However, if you’re working in Go, you may be wondering how to achieve the same functionality. Fortunately, Go provides a similar method called `strconv.Itoa()`.
`strconv.Itoa()` takes an integer as its argument and returns the integer converted to a string. Here’s an example:
package main
import (
"fmt"
"strconv"
)
func main() {
num := 42
str := strconv.Itoa(num)
fmt.Println(str)
}
In this example, we declare an integer variable `num` with a value of 42. We then use `strconv.Itoa()` to convert `num` to a string and assign the result to the variable `str`. Finally, we print the value of `str` to the console.
It’s important to note that `strconv.Itoa()` only works with integers. If you need to convert other data types to strings, such as floats or booleans, you’ll need to use a different method. However, for converting integers to strings, `strconv.Itoa()` is a simple and effective solution.
Equivalent of Java String valueOf in Go
In conclusion, the Go programming language provides a similar function to Java’s String valueOf function, called strconv.Itoa. This function converts an integer to its equivalent string representation. It is a simple and efficient way to convert integers to strings in Go. Additionally, Go also provides other functions in the strconv package for converting other data types to strings, such as strconv.FormatFloat for converting floating-point numbers to strings. Overall, Go’s strconv package provides a robust set of functions for converting data types to strings, making it a powerful tool for developers working with string manipulation in Go.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |