The Java String valueOf function is a method that converts different types of data into a string representation. It can be used to convert primitive data types such as int, float, double, and boolean, as well as objects such as arrays and characters, into a string. The valueOf function returns a string object that represents the specified value. This method is useful when you need to concatenate different types of data into a single string or when you need to convert a non-string value into a string for display or manipulation purposes. Keep reading below to learn how to Java String valueOf in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
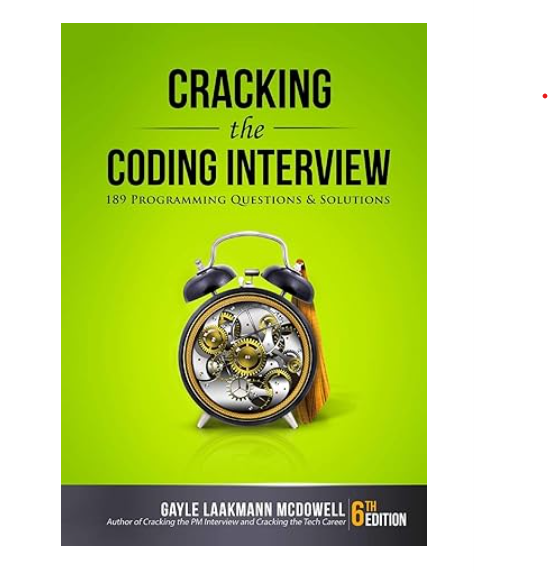
Java String valueOf in Javascript With Example Code
Java’s String class has a method called `valueOf()` that converts different types of data into a string. Similarly, JavaScript also has a `toString()` method that converts a value to a string. However, there may be situations where you need to convert a value to a string in a specific format, which is where the `String()` constructor comes in handy. In this blog post, we will discuss how to use the `String()` constructor to achieve the same functionality as Java’s `valueOf()` method in JavaScript.
To use the `String()` constructor, simply pass the value you want to convert as an argument. For example, if you want to convert a number to a string, you can do the following:
const num = 42;
const str = String(num);
console.log(str); // "42"
Similarly, you can convert a boolean value to a string:
const bool = true;
const str = String(bool);
console.log(str); // "true"
You can also convert an array to a string:
const arr = [1, 2, 3];
const str = String(arr);
console.log(str); // "1,2,3"
Note that the `String()` constructor does not work for objects. If you try to convert an object to a string using the `String()` constructor, you will get the string representation of the object, which is usually not what you want. In such cases, you can use the `JSON.stringify()` method to convert the object to a string in a specific format.
In conclusion, the `String()` constructor in JavaScript can be used to achieve the same functionality as Java’s `valueOf()` method. It is a simple and easy-to-use method that can convert different types of data to a string in a specific format.
Equivalent of Java String valueOf in Javascript
In conclusion, the equivalent function of Java’s String valueOf() in JavaScript is the toString() method. Both functions are used to convert non-string data types into string data types. The toString() method is a built-in function in JavaScript that can be called on any object to return its string representation. It is a versatile function that can be used on numbers, arrays, and even custom objects. By using the toString() method, developers can easily convert data types and manipulate them as strings in their JavaScript code. Overall, the toString() method is a powerful tool that can simplify string manipulation in JavaScript.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |