The JavaScript Array concat function is used to merge two or more arrays into a single array. It does not modify the original arrays, but instead returns a new array that contains all the elements from the original arrays. The concat function can take any number of arguments, each of which can be an array or a value. If the argument is an array, its elements are added to the new array. If the argument is a value, it is added to the new array as a single element. The concat function is useful when you need to combine multiple arrays into a single array, or when you need to add new elements to an existing array without modifying the original array. Keep reading below to learn how to Javascript Array concat in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
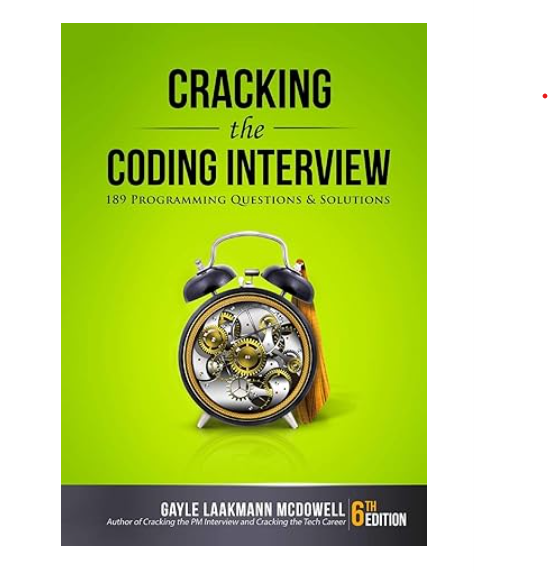
Javascript Array concat in Java With Example Code
JavaScript arrays are a powerful tool for storing and manipulating data in web development. One useful method for working with arrays is the `concat()` method, which allows you to combine two or more arrays into a single array. In this blog post, we will explore how to use the `concat()` method in Java.
To use the `concat()` method in Java, you first need to create two or more arrays that you want to combine. For example, let’s say you have two arrays of numbers:
int[] array1 = {1, 2, 3};
int[] array2 = {4, 5, 6};
To combine these arrays using the `concat()` method, you can create a new array and call the `concat()` method on it, passing in the arrays you want to combine as arguments. Here’s an example:
int[] combinedArray = ArrayUtils.concat(array1, array2);
In this example, we are using the `ArrayUtils` class from the Apache Commons Lang library to concatenate the arrays. If you are not using this library, you can use the built-in `System.arraycopy()` method to achieve the same result:
int[] combinedArray = new int[array1.length + array2.length];
System.arraycopy(array1, 0, combinedArray, 0, array1.length);
System.arraycopy(array2, 0, combinedArray, array1.length, array2.length);
In this example, we are creating a new array with a length equal to the combined length of the two input arrays. We then use the `System.arraycopy()` method to copy the elements from each input array into the new array.
In conclusion, the `concat()` method is a useful tool for combining arrays in Java. Whether you are using the Apache Commons Lang library or the built-in `System.arraycopy()` method, the `concat()` method can help you streamline your code and make it more efficient.
Equivalent of Javascript Array concat in Java
In conclusion, the equivalent Java function for the Javascript Array concat function is the ArrayList addAll() method. This method allows us to concatenate two or more ArrayLists into a single ArrayList. It is important to note that unlike the Javascript Array concat function, the addAll() method does not modify the original ArrayLists, but instead creates a new ArrayList with the concatenated values. Additionally, the addAll() method can only concatenate ArrayLists of the same data type. Overall, the addAll() method is a useful tool for concatenating ArrayLists in Java and can be used in a variety of applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |