The JavaScript Array concat function is used to merge two or more arrays into a single array. It does not modify the original arrays, but instead returns a new array that contains all the elements from the original arrays. The concat function can take any number of arguments, each of which can be an array or a value. If the argument is an array, its elements are added to the new array. If the argument is a value, it is added to the new array as a single element. The concat function is useful when you need to combine multiple arrays into a single array, or when you need to add new elements to an existing array without modifying the original array. Keep reading below to learn how to Javascript Array concat in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
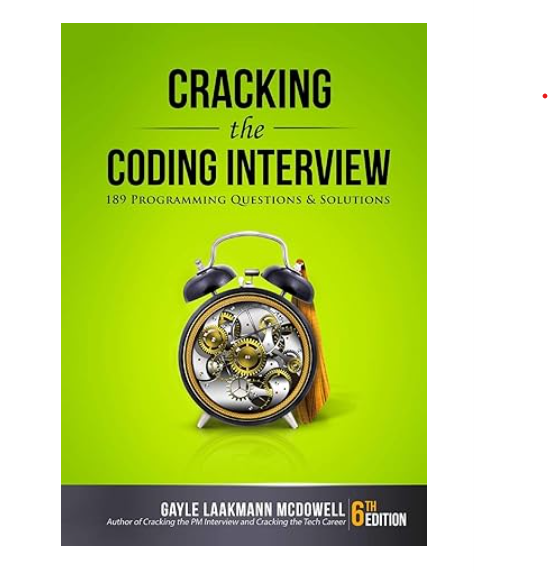
Javascript Array concat in Kotlin With Example Code
JavaScript Array Concat in Kotlin
In Kotlin, you can use the `plus` operator to concatenate two arrays. However, if you want to concatenate more than two arrays, you can use the `plus` operator multiple times or use the `concat` function.
The `concat` function is a member function of the `Array` class in Kotlin. It takes one or more arrays as arguments and returns a new array that contains all the elements of the original arrays.
Here’s an example of using the `concat` function to concatenate three arrays:
val array1 = arrayOf(1, 2, 3)
val array2 = arrayOf(4, 5, 6)
val array3 = arrayOf(7, 8, 9)
val result = array1.concat(array2, array3)
println(result.contentToString()) // Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, we first create three arrays `array1`, `array2`, and `array3`. We then use the `concat` function to concatenate all three arrays into a new array called `result`. Finally, we print the contents of the `result` array using the `contentToString` function.
You can also use the spread operator (`*`) to pass an array as multiple arguments to the `concat` function. Here’s an example:
val array1 = arrayOf(1, 2, 3)
val array2 = arrayOf(4, 5, 6)
val array3 = arrayOf(7, 8, 9)
val result = array1.concat(*array2, *array3)
println(result.contentToString()) // Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, we use the spread operator to pass the `array2` and `array3` arrays as separate arguments to the `concat` function.
In summary, the `concat` function is a useful tool for concatenating arrays in Kotlin. It allows you to easily combine multiple arrays into a single array.
Equivalent of Javascript Array concat in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to concatenate arrays using the `plus` operator. This operator allows developers to easily combine two or more arrays into a single array without the need for complex code or external libraries. Additionally, Kotlin’s `plus` operator can be used with any type of array, including primitive types and custom objects. This makes it a versatile and flexible tool for developers working on a wide range of projects. Overall, the `plus` operator in Kotlin is a great alternative to the equivalent JavaScript Array concat function, and is definitely worth exploring for any Kotlin developer looking to streamline their array manipulation code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |