The JavaScript Array every() function is used to check if all the elements in an array pass a certain test. It takes in a callback function as an argument, which is executed on each element of the array. If the callback function returns true for all elements, then the every() function returns true. If the callback function returns false for any element, then the every() function returns false. The every() function stops executing the callback function as soon as it encounters the first element for which the callback function returns false. The every() function returns true for an empty array. Keep reading below to learn how to Javascript Array every in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
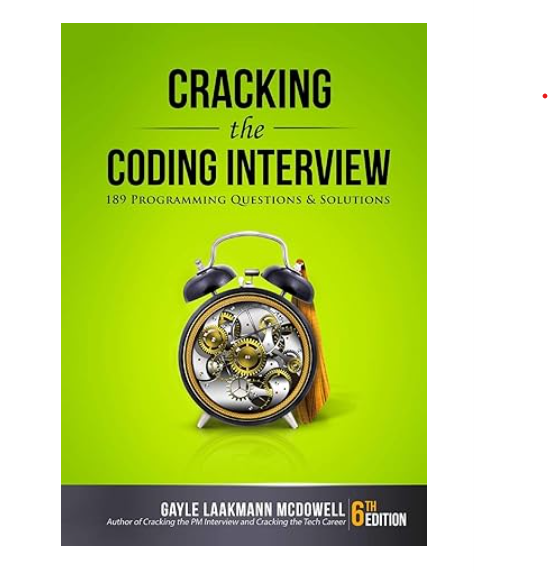
Javascript Array every in C# With Example Code
When working with arrays in C#, you may come across a situation where you need to check if every element in the array meets a certain condition. This is where the Every
method comes in handy.
The Every
method is a LINQ extension method that returns a boolean value indicating whether every element in the array satisfies a given condition. The syntax for using the Every
method is as follows:
bool result = array.Every(element => condition);
Here, array
is the array you want to check, element
is a placeholder for each element in the array, and condition
is the condition that each element must satisfy. The Every
method will iterate through each element in the array and apply the condition to it. If every element satisfies the condition, the method will return true
. Otherwise, it will return false
.
Let’s look at an example. Suppose we have an array of integers and we want to check if every element in the array is greater than 5:
int[] numbers = { 6, 7, 8, 9 };
bool result = numbers.Every(num => num > 5);
In this case, the result
variable will be set to true
because every element in the numbers
array is greater than 5.
It’s important to note that the Every
method will throw an exception if the array is null or empty. To avoid this, you can add a null check or an empty check before calling the method.
Overall, the Every
method is a useful tool for checking if every element in an array meets a certain condition. By using this method, you can easily and efficiently perform this check in your C# code.
Equivalent of Javascript Array every in C#
In conclusion, the equivalent of the Javascript Array every function in C# is the LINQ extension method called “All”. Both functions serve the same purpose of checking if every element in an array meets a certain condition. However, the syntax and implementation differ slightly between the two languages. While the Javascript every function is a built-in method of the Array object, the C# All method is part of the LINQ library and requires an IEnumerable object. Despite these differences, both functions are powerful tools for filtering and manipulating arrays in their respective languages. As a developer, it’s important to understand the similarities and differences between these functions in order to write efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |