The JavaScript Array every() function is used to check if all the elements in an array pass a certain test. It takes in a callback function as an argument, which is executed on each element of the array. If the callback function returns true for all elements, then the every() function returns true. If the callback function returns false for any element, then the every() function returns false. The every() function stops executing the callback function as soon as it encounters the first element for which the callback function returns false. The every() function returns true for an empty array. Keep reading below to learn how to Javascript Array every in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
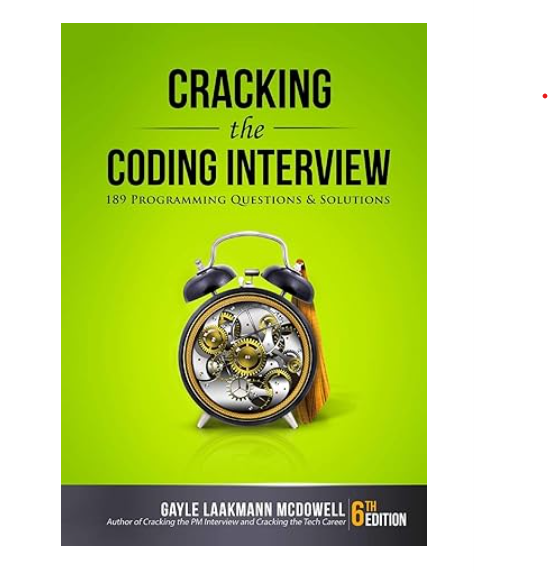
Javascript Array every in C++ With Example Code
JavaScript’s `every()` method is a useful tool for checking if all elements in an array pass a certain test. While C++ does not have a built-in `every()` method, we can create our own implementation using a loop and a boolean variable.
To start, we’ll create a function called `every()` that takes in an array and a test function as parameters. The test function will be used to check if each element in the array passes a certain condition. Here’s what the function signature looks like:
bool every(int arr[], int size, bool (*test)(int));
The `arr` parameter is the array we want to test, `size` is the size of the array, and `test` is a pointer to the test function.
Next, we’ll create the implementation of the `every()` function. We’ll start by creating a boolean variable called `result` and setting it to `true`. We’ll then loop through each element in the array and call the test function on each element. If the test function returns `false` for any element, we’ll set `result` to `false` and break out of the loop. Here’s what the code looks like:
bool every(int arr[], int size, bool (*test)(int)) {
bool result = true;
for (int i = 0; i < size; i++) {
if (!test(arr[i])) {
result = false;
break;
}
}
return result;
}
Now that we have our `every()` function, we can use it to test arrays with different conditions. For example, let's say we have an array of integers and we want to check if all elements are even. We can create a test function that checks if a number is even and pass it into the `every()` function. Here's what the code looks like:
bool isEven(int num) {
return num % 2 == 0;
}
int main() {
int arr[] = {2, 4, 6, 8, 10};
int size = sizeof(arr) / sizeof(arr[0]);
bool allEven = every(arr, size, isEven);
if (allEven) {
std::cout << "All elements are even" << std::endl;
} else {
std::cout << "Not all elements are even" << std::endl;
}
return 0;
}
In this example, the `isEven()` function checks if a number is even by using the modulo operator. We then pass this function into the `every()` function along with our array of integers. The `every()` function returns `true` if all elements in the array are even, and `false` otherwise. We can then use an if statement to print out the appropriate message.
In conclusion, while C++ does not have a built-in `every()` method like JavaScript, we can create our own implementation using a loop and a boolean variable. This allows us to test arrays with different conditions and perform actions based on the results.
Equivalent of Javascript Array every in C++
In conclusion, the equivalent Javascript Array every function in C++ is a powerful tool for developers who want to streamline their code and improve their programming efficiency. By using this function, developers can easily iterate through an array and check if every element meets a certain condition, without having to write lengthy and complex loops. This function is particularly useful for developers who work with large datasets or complex algorithms, as it allows them to quickly and easily filter out unwanted data and focus on the elements that meet their specific criteria. Overall, the equivalent Javascript Array every function in C++ is a valuable addition to any developer's toolkit, and can help to simplify and streamline the coding process.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |