The JavaScript Array every() function is used to check if all the elements in an array pass a certain test. It takes in a callback function as an argument, which is executed on each element of the array. If the callback function returns true for all elements, then the every() function returns true. If the callback function returns false for any element, then the every() function returns false. The every() function stops executing the callback function as soon as it encounters the first element for which the callback function returns false. The every() function returns true for an empty array. Keep reading below to learn how to Javascript Array every in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
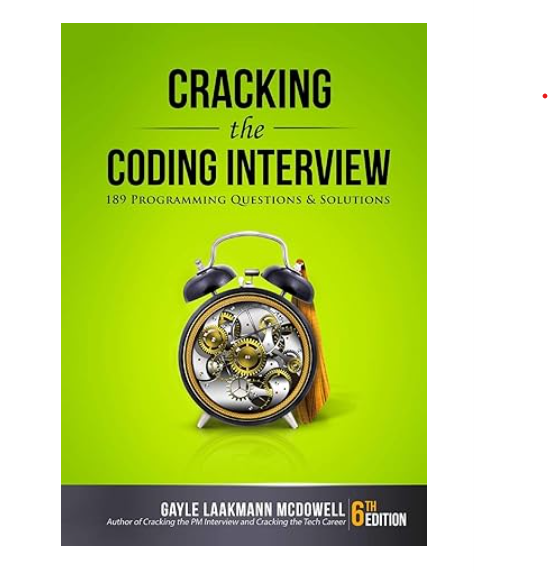
Javascript Array every in Rust With Example Code
JavaScript’s `every` method is a useful tool for checking if every element in an array passes a certain test. Rust, being a systems programming language, doesn’t have a built-in `every` method for arrays. However, we can easily implement our own version of `every` using Rust’s powerful iterator methods.
To implement `every` in Rust, we can use the `Iterator` trait’s `all` method. The `all` method returns `true` if every element in the iterator satisfies a given predicate. We can use this method to check if every element in our array passes our test.
Here’s an example implementation of `every` in Rust:
fn every
where
F: FnMut(&T) -> bool,
{
arr.iter().all(|x| f(x))
}
In this implementation, we take in an array `arr` and a closure `f` that takes in a reference to an element of the array and returns a boolean. We then use the `iter` method to create an iterator over the array and call the `all` method on it with our closure `f`.
Here’s an example usage of our `every` function:
fn main() {
let arr = [1, 2, 3, 4, 5];
let result = every(&arr, |x| *x > 0);
println!("{}", result); // prints "true"
let result = every(&arr, |x| *x > 3);
println!("{}", result); // prints "false"
}
In this example, we create an array `arr` and use our `every` function to check if every element in the array is greater than 0 and greater than 3. The first check returns `true` because every element in the array is greater than 0, while the second check returns `false` because not every element in the array is greater than 3.
With this implementation of `every`, we can easily check if every element in an array passes a certain test in Rust.
Equivalent of Javascript Array every in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to work with arrays through its equivalent functions to those found in JavaScript. With Rust’s built-in array methods, developers can easily manipulate and iterate through arrays, making it a great choice for performance-critical applications. Additionally, Rust’s strong type system and memory safety features ensure that arrays are handled in a safe and reliable manner. Whether you’re a seasoned developer or just starting out, Rust’s array functions provide a great way to work with arrays in a fast and efficient manner.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |