The JavaScript Array filter function is a built-in method that allows you to create a new array with all elements that pass a certain test. It takes a callback function as an argument, which is executed on each element of the array. The callback function should return a boolean value, indicating whether the element should be included in the new array or not. The filter function then returns a new array containing only the elements that passed the test. This method is useful for filtering out unwanted data from an array, or for creating a new array with specific criteria. Keep reading below to learn how to Javascript Array filter in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
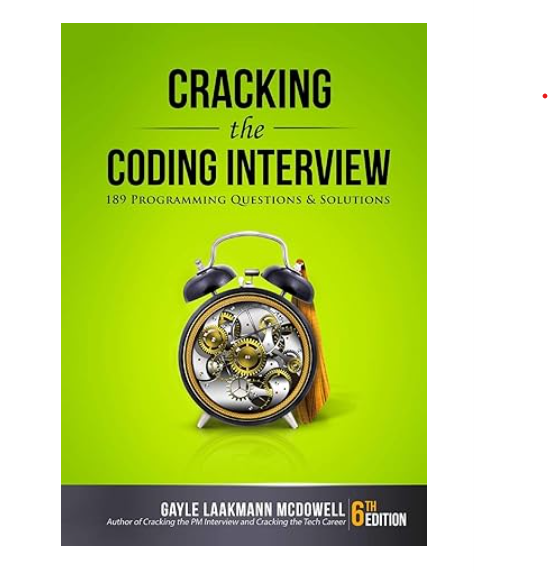
Javascript Array filter in Java With Example Code
JavaScript Array filter is a method that allows you to filter out elements from an array based on a condition. In this blog post, we will discuss how to use the filter method in Java.
To use the filter method in Java, you first need to create an array. Here is an example:
int[] numbers = {1, 2, 3, 4, 5};
Now, let’s say we want to filter out all the even numbers from this array. We can use the filter method to achieve this. Here is how:
int[] evenNumbers = Arrays.stream(numbers).filter(n -> n % 2 == 0).toArray();
In the above code, we are using the filter method to filter out all the even numbers from the array. The filter method takes a lambda expression as an argument, which is used to test each element in the array. In this case, we are testing if the element is even or not.
The filter method returns a stream of elements that pass the test. We then convert this stream back to an array using the toArray method.
Now, let’s print out the even numbers array to see the result:
System.out.println(Arrays.toString(evenNumbers));
This will output:
[2, 4]
As you can see, the filter method has successfully filtered out all the even numbers from the original array.
In conclusion, the filter method in Java is a powerful tool that allows you to filter out elements from an array based on a condition. It is easy to use and can save you a lot of time and effort when working with arrays.
Equivalent of Javascript Array filter in Java
In conclusion, the equivalent Java function for the Javascript Array filter function is the Stream API’s filter() method. This method allows developers to filter elements in a collection based on a given predicate. It is a powerful tool that can be used to simplify code and improve performance. By using the filter() method, developers can easily manipulate collections in Java, just like they would in Javascript. Overall, the filter() method is a great addition to the Java language and is a must-know for any Java developer looking to improve their coding skills.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |