The JavaScript Array filter function is a built-in method that allows you to create a new array with all elements that pass a certain test. It takes a callback function as an argument, which is executed on each element of the array. The callback function should return a boolean value, indicating whether the element should be included in the new array or not. The filter function then returns a new array containing only the elements that passed the test. This method is useful for filtering out unwanted data from an array, or for creating a new array with specific criteria. Keep reading below to learn how to Javascript Array filter in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
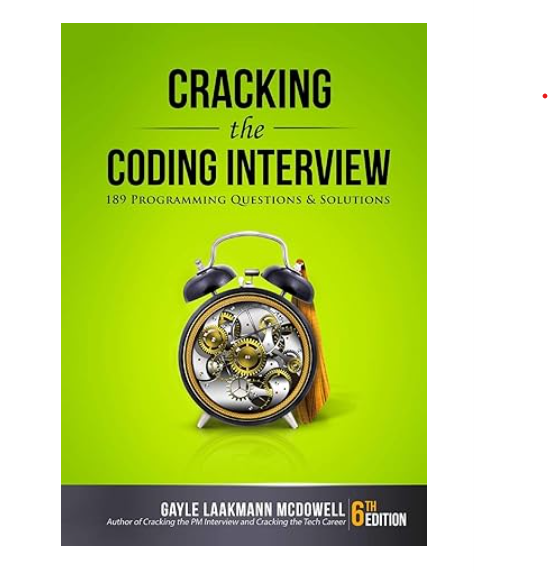
Javascript Array filter in Kotlin With Example Code
JavaScript Array filter is a powerful method that allows you to filter out elements from an array based on a condition. Kotlin, being a versatile language, also provides a similar method to filter arrays. In this blog post, we will explore how to use the filter method in Kotlin.
To use the filter method in Kotlin, you first need to have an array. Let’s say we have an array of numbers:
val numbers = arrayOf(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
Now, let’s say we want to filter out all the even numbers from this array. We can use the filter method to achieve this:
val evenNumbers = numbers.filter { it % 2 == 0 }
In the above code, we are using the filter method on the numbers array. The filter method takes a lambda expression as an argument, which is used to define the condition for filtering. In this case, we are checking if the number is even or not by using the modulo operator (%).
The filter method returns a new array that contains only the elements that satisfy the condition. In this case, the evenNumbers array will contain [2, 4, 6, 8, 10].
You can also use the filter method to filter out elements based on other conditions. For example, let’s say we have an array of strings:
val names = arrayOf("John", "Jane", "Bob", "Alice", "Mike")
Now, let’s say we want to filter out all the names that start with the letter “J”. We can use the filter method to achieve this:
val filteredNames = names.filter { it.startsWith("J") }
In the above code, we are using the filter method on the names array. The filter method takes a lambda expression as an argument, which is used to define the condition for filtering. In this case, we are checking if the name starts with the letter “J” or not by using the startsWith method.
The filter method returns a new array that contains only the elements that satisfy the condition. In this case, the filteredNames array will contain [“John”, “Jane”].
In conclusion, the filter method in Kotlin is a powerful tool that allows you to filter out elements from an array based on a condition. It is easy to use and can be used to filter out elements based on various conditions.
Equivalent of Javascript Array filter in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to filter arrays using the `filter()` function. This function works similarly to the equivalent JavaScript Array filter function, allowing developers to easily manipulate and filter arrays based on specific criteria. With Kotlin’s concise syntax and functional programming capabilities, filtering arrays has never been easier. Whether you’re a seasoned developer or just starting out, the `filter()` function in Kotlin is a valuable tool to have in your programming arsenal. So, if you’re looking for a more efficient way to filter arrays in your Kotlin projects, be sure to give the `filter()` function a try.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |