The JavaScript Array filter function is a built-in method that allows you to create a new array with all elements that pass a certain test. It takes a callback function as an argument, which is executed on each element of the array. The callback function should return a boolean value, indicating whether the element should be included in the new array or not. The filter function then returns a new array containing only the elements that passed the test. This method is useful for filtering out unwanted data from an array, or for creating a new array with specific criteria. Keep reading below to learn how to Javascript Array filter in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
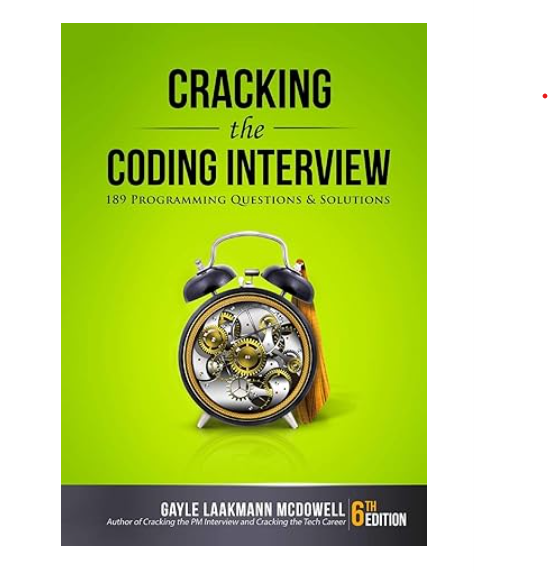
Javascript Array filter in PHP With Example Code
JavaScript Array filter is a powerful method that allows you to filter out elements from an array based on a certain condition. This method is not available in PHP by default, but you can easily implement it using a custom function. In this blog post, we will show you how to implement JavaScript Array filter in PHP.
To implement JavaScript Array filter in PHP, you can create a custom function that takes an array and a callback function as arguments. The callback function should return true or false based on a certain condition. The custom function should then loop through the array and call the callback function for each element. If the callback function returns true, the element is added to a new array. If the callback function returns false, the element is skipped.
Here is an example code snippet that demonstrates how to implement JavaScript Array filter in PHP:
function array_filter_js($array, $callback) {
$filtered_array = array();
foreach ($array as $element) {
if ($callback($element)) {
$filtered_array[] = $element;
}
}
return $filtered_array;
}
$numbers = array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
$even_numbers = array_filter_js($numbers, function($number) {
return $number % 2 == 0;
});
print_r($even_numbers);
In this example, we have created a custom function called array_filter_js that takes an array and a callback function as arguments. We have also created an array of numbers from 1 to 10.
We then call the array_filter_js function and pass in the numbers array and a callback function that checks if a number is even. The callback function returns true if the number is even and false if it is odd.
The array_filter_js function loops through the numbers array and calls the callback function for each element. If the callback function returns true, the element is added to a new array called $filtered_array. If the callback function returns false, the element is skipped.
Finally, we print out the $even_numbers array, which contains only the even numbers from the original $numbers array.
In conclusion, implementing JavaScript Array filter in PHP is a simple process that can be done using a custom function. By using this method, you can easily filter out elements from an array based on a certain condition.
Equivalent of Javascript Array filter in PHP
In conclusion, the equivalent of the Javascript Array filter function in PHP is the array_filter() function. This function allows developers to filter an array based on a given condition and return a new array with the filtered values. The array_filter() function is a powerful tool for manipulating arrays in PHP and can be used in a variety of applications. Whether you are working on a small project or a large-scale application, the array_filter() function can help you streamline your code and improve the efficiency of your program. So, if you are looking for a way to filter arrays in PHP, be sure to give the array_filter() function a try.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |