The JavaScript Array filter function is a built-in method that allows you to create a new array with all elements that pass a certain test. It takes a callback function as an argument, which is executed on each element of the array. The callback function should return a boolean value, indicating whether the element should be included in the new array or not. The filter function then returns a new array containing only the elements that passed the test. This method is useful for filtering out unwanted data from an array, or for creating a new array with specific criteria. Keep reading below to learn how to Javascript Array filter in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
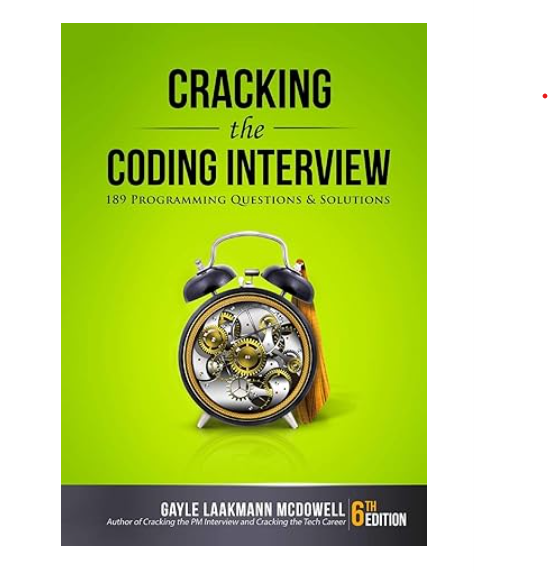
Javascript Array filter in Rust With Example Code
JavaScript is a popular programming language used for web development. One of its useful features is the ability to filter arrays using the `filter()` method. Rust is another programming language that has gained popularity in recent years. In this blog post, we will explore how to filter arrays in Rust using the `filter()` method.
To filter an array in Rust, we first need to create an array. Here is an example array:
let numbers = [1, 2, 3, 4, 5];
To filter this array, we can use the `filter()` method. The `filter()` method takes a closure as an argument and returns a new array containing only the elements that satisfy the closure. Here is an example of using the `filter()` method to filter the `numbers` array:
let filtered_numbers = numbers.iter().filter(|&n| n % 2 == 0).collect::
In this example, we are filtering the `numbers` array to only include even numbers. The closure `|&n| n % 2 == 0` checks if the number is even by checking if the remainder of the number divided by 2 is 0. The `collect()` method is used to collect the filtered elements into a new array.
We can also use the `filter()` method to filter arrays of structs. Here is an example struct:
struct Person {
name: String,
age: u32,
}
We can create an array of `Person` structs and filter it based on the age of the person. Here is an example:
let people = vec![
Person { name: "Alice".to_string(), age: 25 },
Person { name: "Bob".to_string(), age: 30 },
Person { name: "Charlie".to_string(), age: 20 },
];
let filtered_people = people.iter().filter(|&p| p.age > 25).collect::
In this example, we are filtering the `people` array to only include people over the age of 25. The closure `|&p| p.age > 25` checks if the age of the person is greater than 25. The `collect()` method is used to collect the filtered elements into a new array.
In conclusion, the `filter()` method in Rust is a powerful tool for filtering arrays and arrays of structs. By using closures, we can filter arrays based on any condition we want.
Equivalent of Javascript Array filter in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to filter arrays using the `iter()` method and the `filter()` function. This equivalent to the JavaScript Array filter function allows developers to easily manipulate and filter arrays in Rust, making it a great choice for data-intensive applications. With Rust’s focus on performance and safety, developers can rest assured that their code will run smoothly and reliably. Whether you’re a seasoned Rust developer or just getting started, the `filter()` function is a valuable tool to have in your arsenal. So why not give it a try and see how it can improve your Rust programming experience?
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |