The JavaScript Array filter function is a built-in method that allows you to create a new array with all elements that pass a certain test. It takes a callback function as an argument, which is executed on each element of the array. The callback function should return a boolean value, indicating whether the element should be included in the new array or not. The filter function then returns a new array containing only the elements that passed the test. This method is useful for filtering out unwanted data from an array, or for creating a new array with specific criteria. Keep reading below to learn how to Javascript Array filter in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
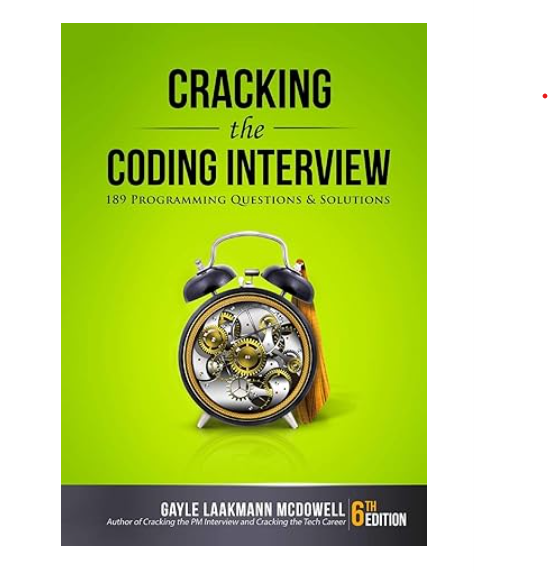
Javascript Array filter in TypeScript With Example Code
JavaScript Array filter is a powerful method that allows you to filter out elements from an array based on a condition. TypeScript, being a superset of JavaScript, also supports this method. In this blog post, we will explore how to use the filter method in TypeScript.
To use the filter method in TypeScript, you first need to define an array. Let’s say we have an array of numbers:
const numbers: number[] = [1, 2, 3, 4, 5];
Now, let’s say we want to filter out all the even numbers from this array. We can use the filter method to achieve this:
const evenNumbers = numbers.filter((num) => num % 2 === 0);
In the above code, we are using an arrow function to define the condition for filtering. The filter method will iterate over each element in the array and pass it to this function. If the function returns true, the element will be included in the filtered array, otherwise, it will be excluded.
In this case, we are checking if the number is even by using the modulo operator (%). If the remainder of the number divided by 2 is 0, it means the number is even and we return true, otherwise, we return false.
We can also use the filter method to filter out elements based on other conditions. For example, let’s say we have an array of objects representing people:
const people = [
{ name: 'John', age: 25 },
{ name: 'Jane', age: 30 },
{ name: 'Bob', age: 20 },
{ name: 'Alice', age: 35 },
];
Now, let’s say we want to filter out all the people who are older than 30. We can use the filter method to achieve this:
const olderThan30 = people.filter((person) => person.age > 30);
In the above code, we are using the arrow function to check if the age of the person is greater than 30. If it is, we return true and the person will be included in the filtered array.
In conclusion, the filter method is a powerful tool that allows you to filter out elements from an array based on a condition. TypeScript fully supports this method, and you can use it to filter out elements from arrays of any type.
Equivalent of Javascript Array filter in TypeScript
In conclusion, the TypeScript Array filter function is a powerful tool that allows developers to filter out unwanted elements from an array based on a given condition. It works in a similar way to the JavaScript Array filter function, but with the added benefit of type safety and improved code readability. By using TypeScript, developers can write more robust and maintainable code that is less prone to errors and easier to understand. Whether you are a seasoned developer or just starting out, the TypeScript Array filter function is a valuable tool to have in your arsenal. So, next time you need to filter an array in TypeScript, give the filter function a try and see how it can simplify your code and improve your productivity.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |