The JavaScript Array forEach function is a built-in method that allows you to iterate over an array and execute a provided function for each element in the array. It takes a callback function as an argument, which is executed once for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The forEach function does not return anything, but it can be used to modify the original array or perform other operations on each element. It is a useful tool for performing repetitive tasks on arrays in a concise and readable way. Keep reading below to learn how to Javascript Array forEach in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
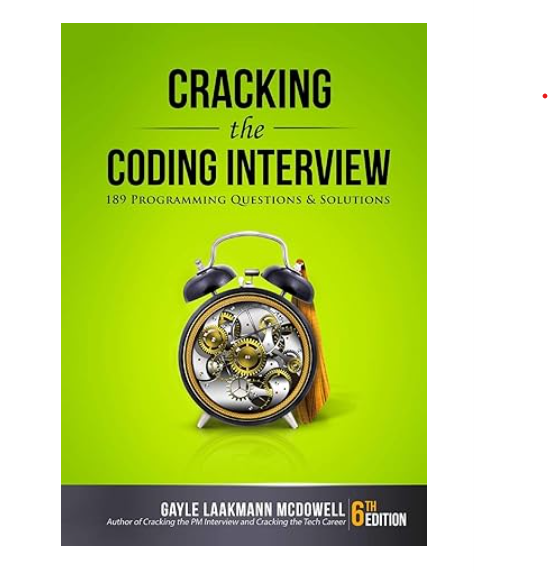
Javascript Array forEach in C++ With Example Code
JavaScript is a popular programming language that is widely used for web development. One of the most commonly used features of JavaScript is the Array forEach method. This method allows you to iterate over an array and perform a specific action on each element. If you are a C++ developer, you may be wondering if there is a similar method available in C++. In this blog post, we will explore how to use the forEach method in C++.
The forEach method in JavaScript takes a callback function as an argument. This function is called for each element in the array and is passed the current element, the index of the element, and the array itself as arguments. The callback function can then perform any action on the element, such as printing it to the console or modifying it.
In C++, there is no built-in forEach method for arrays. However, you can achieve similar functionality using a for loop. Here is an example of how to iterate over an array in C++ using a for loop:
int arr[] = {1, 2, 3, 4, 5};
int length = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < length; i++) { // Perform action on arr[i] }
In this example, we first define an array of integers and calculate its length. We then use a for loop to iterate over the array and perform an action on each element. You can replace the comment with any action you want to perform on the element.
While this method may not be as concise as the JavaScript forEach method, it provides similar functionality and is easy to understand for C++ developers. With this knowledge, you can now iterate over arrays in C++ and perform any action you need to on each element.
Equivalent of Javascript Array forEach in C++
In conclusion, the equivalent of the Javascript Array forEach function in C++ is the std::for_each function. This function allows us to iterate over a range of elements in a container and apply a function to each element. It provides a simple and efficient way to perform operations on each element of an array or vector in C++. By using the std::for_each function, we can easily apply a function to each element of a container without having to write a loop ourselves. This saves us time and effort, and makes our code more concise and readable. Overall, the std::for_each function is a powerful tool for iterating over containers in C++, and is a great alternative to traditional for loops.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |