The JavaScript Array forEach function is a built-in method that allows you to iterate over an array and execute a provided function once for each element in the array. It takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The forEach function does not return anything, but it can be used to modify the original array or perform other operations on each element. It is a useful tool for performing operations on arrays in a concise and readable way. Keep reading below to learn how to Javascript Array forEach in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
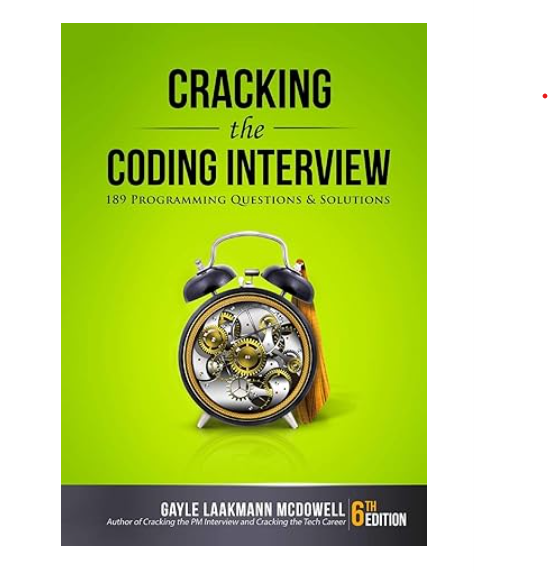
Javascript Array forEach in Kotlin With Example Code
JavaScript is a popular programming language used for web development. One of its most commonly used features is the Array forEach method. Kotlin, a programming language that runs on the Java Virtual Machine, also has a forEach method for arrays. In this blog post, we will explore how to use the JavaScript Array forEach method in Kotlin.
To use the JavaScript Array forEach method in Kotlin, we first need to create an array. We can do this using the arrayOf function:
val numbers = arrayOf(1, 2, 3, 4, 5)
Now that we have an array, we can use the forEach method to iterate over each element in the array. The forEach method takes a lambda expression as its argument. The lambda expression is executed for each element in the array:
numbers.forEach { println(it) }
In this example, the lambda expression simply prints each element in the array to the console. The it
keyword refers to the current element being iterated over.
We can also use the forEach method to modify the elements in the array. For example, let’s say we want to double each element in the array:
numbers.forEach { it * 2 }
In this example, the lambda expression multiplies each element by 2. However, this code doesn’t actually modify the original array. To modify the original array, we need to use the index of the current element:
numbers.forEachIndexed { index, value -> numbers[index] = value * 2 }
In this example, the forEachIndexed method is used instead of the forEach method. The forEachIndexed method takes a lambda expression with two arguments: the index of the current element and the value of the current element. We use the index to modify the value of the current element in the original array.
In conclusion, the JavaScript Array forEach method can be used in Kotlin to iterate over arrays and modify their elements. By using the lambda expression argument, we can execute code for each element in the array. The forEachIndexed method can be used to modify the original array.
Equivalent of Javascript Array forEach in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to iterate over arrays using the forEach function. This function is similar to the JavaScript Array forEach function, but with some added benefits such as type safety and null safety. With the forEach function, developers can easily loop through arrays and perform operations on each element without having to write complex for loops. Additionally, the forEach function can be used with lambdas, making it even more versatile and flexible. Overall, the forEach function in Kotlin is a valuable tool for any developer looking to streamline their array iteration process.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |