The JavaScript Array forEach function is a built-in method that allows you to iterate over an array and execute a provided function once for each element in the array. It takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The forEach function does not return anything, but it can be used to modify the original array or perform other operations on each element. It is a useful tool for performing operations on arrays in a concise and readable way. Keep reading below to learn how to Javascript Array forEach in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
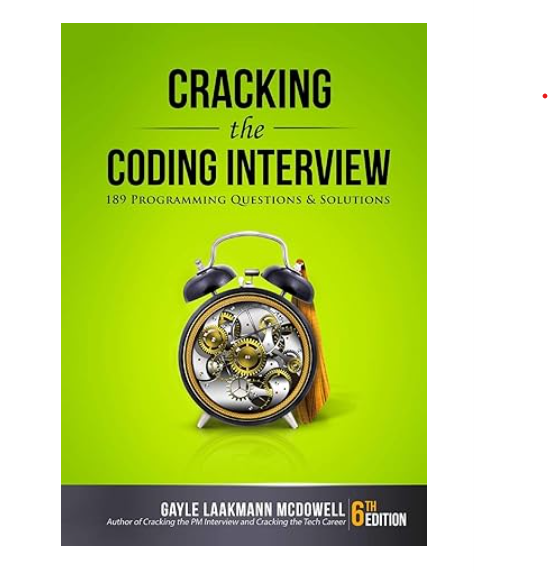
Javascript Array forEach in PHP With Example Code
JavaScript is a popular programming language used for creating interactive web pages. One of the most commonly used features of JavaScript is the Array forEach method. This method allows you to loop through each element of an array and perform a specific action on each element. In this blog post, we will explore how to use the JavaScript Array forEach method in PHP.
To use the JavaScript Array forEach method in PHP, we need to first understand how it works in JavaScript. The forEach method takes a callback function as an argument. This callback function is executed for each element in the array. The callback function takes three arguments: the current element, the index of the current element, and the array itself.
Here is an example of how to use the JavaScript Array forEach method in PHP:
$numbers = array(1, 2, 3, 4, 5);
foreach ($numbers as $number) {
echo $number;
}
In this example, we have an array of numbers and we are using a foreach loop to loop through each element of the array and print it to the screen. This is a basic example, but it demonstrates how to loop through an array in PHP.
Now, let’s see how we can use the JavaScript Array forEach method in PHP. We can use the array_walk function in PHP to achieve the same result as the JavaScript Array forEach method. The array_walk function takes two arguments: the array to be walked and the callback function to be executed for each element.
Here is an example of how to use the JavaScript Array forEach method in PHP using the array_walk function:
$numbers = array(1, 2, 3, 4, 5);
function printNumber($number, $key) {
echo $number;
}
array_walk($numbers, 'printNumber');
In this example, we have defined a callback function called printNumber that takes two arguments: the current element and the index of the current element. We then use the array_walk function to loop through each element of the array and execute the printNumber function for each element.
In conclusion, the JavaScript Array forEach method is a powerful tool for looping through arrays in JavaScript. While PHP does not have a built-in forEach method, we can use the array_walk function to achieve the same result. By understanding how the JavaScript Array forEach method works, we can use it in PHP to make our code more efficient and easier to read.
Equivalent of Javascript Array forEach in PHP
In conclusion, the equivalent of the Javascript Array forEach function in PHP is the foreach loop. This loop allows developers to iterate through an array and perform actions on each element without the need for a counter or index. The foreach loop is a powerful tool in PHP that can simplify code and make it more readable. It is important to note that the syntax for the foreach loop in PHP is slightly different than in Javascript, but the concept remains the same. By understanding the similarities and differences between these two languages, developers can write efficient and effective code in both environments.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |