The JavaScript Array forEach function is a built-in method that allows you to iterate over an array and execute a provided function for each element in the array. It takes a callback function as an argument, which is executed once for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The forEach function does not return anything, but it can be used to modify the original array or perform other operations on each element. It is a useful tool for performing repetitive tasks on arrays in a concise and readable way. Keep reading below to learn how to Javascript Array forEach in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
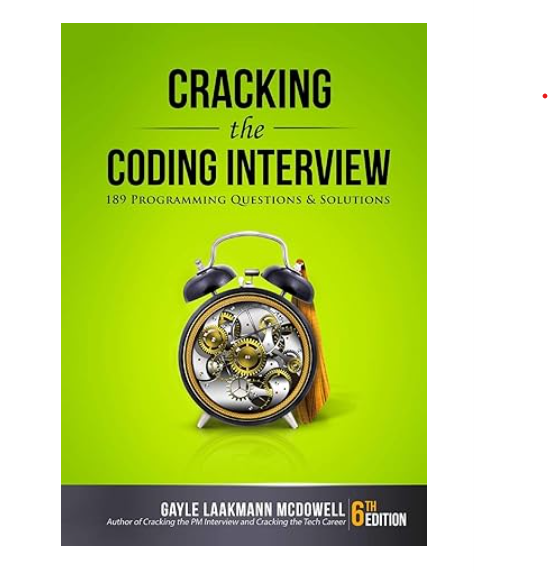
Javascript Array forEach in Python With Example Code
JavaScript is a popular programming language used for web development. One of its useful features is the `forEach` method for arrays. This method allows you to iterate over each element in an array and perform a function on it. Python, another popular programming language, does not have a built-in `forEach` method for arrays. However, there are ways to achieve similar functionality in Python.
One way to iterate over an array in Python is to use a `for` loop. Here’s an example:
my_array = [1, 2, 3, 4, 5]
for element in my_array:
print(element)
This code will output each element in the `my_array` array.
Another way to achieve similar functionality to `forEach` in Python is to use a `map` function. The `map` function applies a function to each element in an iterable and returns a new iterable with the results. Here’s an example:
my_array = [1, 2, 3, 4, 5]
def my_function(element):
return element * 2
new_array = list(map(my_function, my_array))
print(new_array)
This code will output a new array with each element in `my_array` multiplied by 2.
While Python does not have a built-in `forEach` method for arrays, there are ways to achieve similar functionality using `for` loops and `map` functions.
Equivalent of Javascript Array forEach in Python
In conclusion, the equivalent function to the Javascript Array forEach in Python is the for loop. While the forEach function in Javascript allows for a more concise and readable code, the for loop in Python provides more flexibility and control over the iteration process. It is important to note that both functions serve the same purpose of iterating over an array and executing a function for each element. As a developer, it is essential to understand the differences between these two functions and choose the one that best suits the specific needs of the project. Ultimately, the choice between the forEach function in Javascript and the for loop in Python will depend on the individual preferences and requirements of the developer.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |