The JavaScript Array forEach function is a built-in method that allows you to iterate over an array and execute a provided function once for each element in the array. It takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The forEach function does not return anything, but it can be used to modify the original array or perform other operations on each element. It is a useful tool for performing operations on arrays in a concise and readable way. Keep reading below to learn how to Javascript Array forEach in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
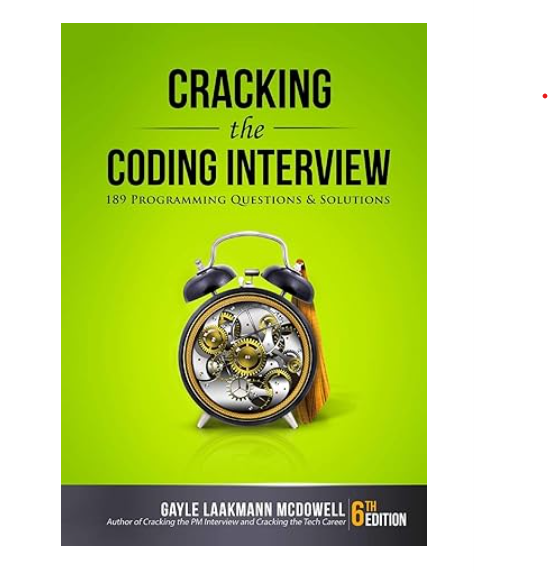
Javascript Array forEach in Rust With Example Code
JavaScript developers who are new to Rust may be wondering how to use the `forEach` method on arrays in Rust. Fortunately, Rust provides a similar method called `iter()` that can be used to iterate over arrays.
To use `iter()` on an array, first create an array and assign it to a variable:
let my_array = [1, 2, 3, 4, 5];
Then, call the `iter()` method on the array:
my_array.iter()
This will return an iterator that can be used to loop over the array. To loop over the array and print each element to the console, use a `for` loop:
for element in my_array.iter() {
println!("{}", element);
}
This will print each element of the array to the console.
Alternatively, you can use the `for_each()` method on the iterator to perform an action on each element of the array:
my_array.iter().for_each(|element| {
println!("{}", element);
});
This will also print each element of the array to the console.
In summary, to iterate over an array in Rust, use the `iter()` method to create an iterator and then use a `for` loop or the `for_each()` method to loop over the iterator and perform an action on each element of the array.
Equivalent of Javascript Array forEach in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to iterate over arrays using the equivalent of the JavaScript Array forEach function. The Rust implementation, called the “for_each” method, allows developers to easily loop through arrays and perform operations on each element without the need for complex syntax or additional libraries. This feature is particularly useful for developers who are transitioning from JavaScript to Rust, as it provides a familiar and intuitive way to work with arrays. Overall, the Rust for_each method is a valuable tool for any developer looking to optimize their array iteration process and improve the performance of their Rust applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |