The JavaScript Array forEach function is a built-in method that allows you to iterate over an array and execute a provided function once for each element in the array. It takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The forEach function does not return anything, but it can be used to modify the original array or perform other operations on each element. It is a useful tool for performing operations on arrays in a concise and readable way. Keep reading below to learn how to Javascript Array forEach in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
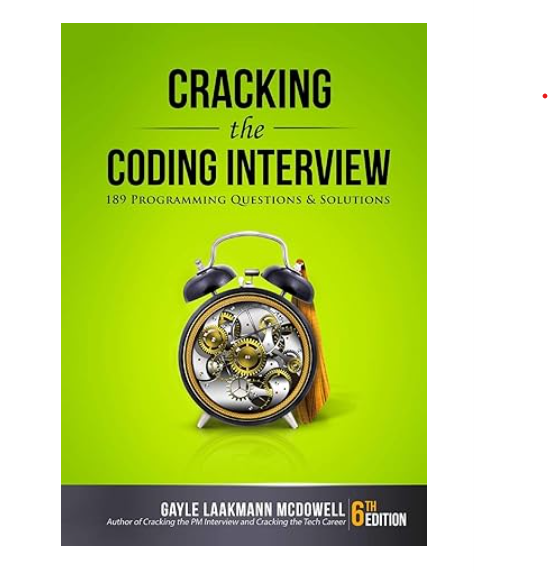
Javascript Array forEach in TypeScript With Example Code
JavaScript is a popular programming language used for web development. TypeScript is a superset of JavaScript that adds optional static typing and other features to the language. In this blog post, we will discuss how to use the JavaScript Array forEach method in TypeScript.
The forEach method is used to iterate over an array and execute a function for each element in the array. In TypeScript, we can use the forEach method on an array of any type. Here is an example of using the forEach method on an array of strings:
const names: string[] = ['Alice', 'Bob', 'Charlie'];
names.forEach((name: string) => {
console.log(name);
});
In this example, we define an array of strings called names. We then call the forEach method on the names array and pass in a function that takes a string parameter called name. The function simply logs the name to the console.
We can also use the forEach method on an array of numbers:
const numbers: number[] = [1, 2, 3];
numbers.forEach((number: number) => {
console.log(number * 2);
});
In this example, we define an array of numbers called numbers. We then call the forEach method on the numbers array and pass in a function that takes a number parameter called number. The function multiplies the number by 2 and logs the result to the console.
In conclusion, the forEach method is a useful tool for iterating over arrays in TypeScript. It can be used on arrays of any type and allows us to execute a function for each element in the array.
Equivalent of Javascript Array forEach in TypeScript
In conclusion, the TypeScript language provides developers with a powerful toolset for building robust and scalable applications. One of the most useful features of TypeScript is its support for the JavaScript Array forEach function. This function allows developers to iterate over arrays and perform operations on each element in a concise and readable manner. By using TypeScript’s type annotations and other language features, developers can write more maintainable and error-free code. Whether you are building a small project or a large-scale application, the TypeScript Array forEach function is an essential tool that can help you write better code and improve your productivity.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |